curl发送post请求用python代码实现
时间: 2023-11-08 18:03:49 浏览: 237
您可以使用Python的requests库来发送POST请求。以下是一个示例代码:
```python
import requests
url = "http://example.com/api" # 请求的URL
data = {"key1": "value1", "key2": "value2"} # 请求的数据
response = requests.post(url, data=data)
print(response.text) # 打印响应内容
```
在这个示例中,您需要将`url`替换为您要发送请求的URL,将`data`替换为您要发送的数据。`requests.post()`方法会发送POST请求并返回一个Response对象,您可以通过调用`response.text`来获取响应内容。
注意:如果需要发送JSON数据而不是表单数据,可以使用`json`参数来发送JSON数据。例如:
```python
import requests
import json
url = "http://example.com/api" # 请求的URL
data = {"key1": "value1", "key2": "value2"} # 请求的数据
response = requests.post(url, json=data)
print(response.text) # 打印响应内容
```
相关问题
python curl转换python请求
在Python中,如果你熟悉使用cURL命令行工具发送HTTP请求,可以使用`requests`库来实现类似的功能。cURL是一个强大的网络通信工具,而`requests`则是一个方便易用的Python库,它封装了底层的HTTP请求。
如果你想要将cURL命令转换成Python `requests`的代码,你可以这样做:
例如,一个基本的cURL命令可能是这样的:
```
curl -X GET "https://api.example.com/data"
```
对应的Python `requests`代码是:
```python
import requests
response = requests.get("https://api.example.com/data")
data = response.json() # 如果返回的是JSON数据
```
在这个例子中,`requests.get()`函数模拟了GET请求,返回的是一个`Response`对象,你可以从中获取到服务器的响应数据(如状态码、响应头和内容等)。
如果你想发送POST请求或携带自定义headers,可以这样做:
```python
headers = {'Content-Type': 'application/json'}
payload = {'key': 'value'}
response = requests.post('https://api.example.com/data', json=payload, headers=headers)
```
python怎么将curl转换成请求代码
在Python中,你可以使用requests库来模拟curl命令发送HTTP请求。curl是一个强大的工具,而requests提供了一个更简单、易读的方式来编写网络请求。如果你有一个cURL命令,可以按照以下步骤将其转换为Python requests代码:
首先,确保已安装`requests`库,如果没有,可以使用pip安装:
```bash
pip install requests
```
然后,假设你有如下的cURL命令示例:
```shell
curl -X GET "https://api.example.com/data" -H "Authorization: Bearer your_token"
```
对应的Python代码会是这样的:
```python
import requests
headers = {
'Authorization': 'Bearer your_token',
}
response = requests.get("https://api.example.com/data", headers=headers)
# 检查响应状态码
if response.status_code == 200:
data = response.json()
print(data)
else:
print(f"请求失败: {response.status_code}")
```
在这个例子中,我们设置了头信息(headers),并使用`get`函数发送GET请求到指定的URL。如果需要其他类型的请求(如POST、PUT等),只需替换`get`为相应的函数,如`post`或`put`。
阅读全文
相关推荐
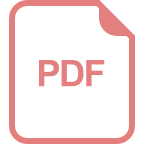
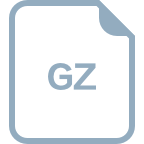
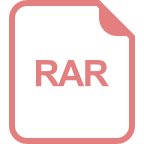
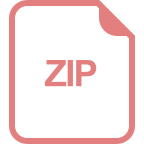
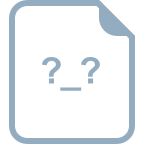
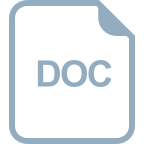
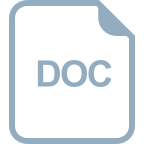









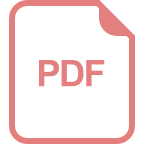