删除单链表相同元素c++
时间: 2024-12-02 18:19:19 浏览: 15
删除单链表中所有相同元素的C++实现通常涉及遍历链表和使用指针技巧。这里是一个简单的示例:
```cpp
#include <iostream>
using namespace std;
struct ListNode {
int val;
ListNode *next;
ListNode(int x) : val(x), next(NULL) {}
};
ListNode* deleteDuplicates(ListNode* head) {
if (head == nullptr || head->next == nullptr) {
return head; // 如果链表只有一个节点或为空,直接返回
}
ListNode dummy(0); // 创建虚拟头节点,避免处理边界条件
dummy.next = head;
ListNode* current = &dummy;
while (current->next != nullptr && current->next->next != nullptr) {
if (current->next->val == current->next->next->val) {
current->next = current->next->next;
} else {
current = current->next;
}
}
return dummy.next; // 返回去除了重复元素的新链表头节点
}
// 测试函数
void printList(ListNode* node) {
while (node != nullptr) {
cout << node->val << " ";
node = node->next;
}
cout << endl;
}
int main() {
ListNode* list = new ListNode(1);
list->next = new ListNode(2);
list->next->next = new ListNode(2);
list->next->next->next = new ListNode(3);
list->next->next->next->next = new ListNode(4);
cout << "Original List: ";
printList(list);
list = deleteDuplicates(list);
cout << "List after removing duplicates: ";
printList(list);
return 0;
}
```
在这个代码里,我们创建了一个`deleteDuplicates`函数,它会遍历链表并删除连续的重复值。如果遇到相同的值,就跳过下一个节点。最后返回新链表的头节点。
阅读全文
相关推荐


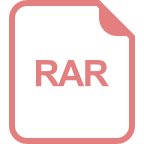















