python 接口回调
时间: 2023-10-19 19:34:35 浏览: 92
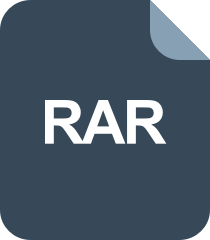
理解接口回调
接口回调是一种常见的编程模式,用于实现程序之间的通信和交互。在Python中,可以通过函数或者类方法来实现接口回调。
如果使用函数来实现接口回调,可以将一个函数作为参数传递给另一个函数或者类的方法,然后在适当的时候调用这个函数来进行回调操作。
示例代码如下:
```python
def callback_function(arg1, arg2):
# 执行回调操作
print("Callback function called with arguments:", arg1, arg2)
def perform_operation(callback):
# 进行一些操作
result = 42
# 调用回调函数
callback("arg1", "arg2")
# 使用回调函数
perform_operation(callback_function)
```
上述代码中,`perform_operation` 函数接收一个回调函数作为参数,并在适当的时候调用这个回调函数。在这个例子中,我们调用 `perform_operation` 函数时会执行回调函数 `callback_function`,并传递两个参数 `"arg1"` 和 `"arg2"`。
如果使用类方法来实现接口回调,可以定义一个接口类,其中包含一个或多个方法,然后创建一个实现该接口的类,并将其实例传递给另一个类的方法进行回调操作。
示例代码如下:
```python
class CallbackInterface:
def callback_method(self, arg1, arg2):
# 执行回调操作
print("Callback method called with arguments:", arg1, arg2)
class PerformOperation:
def __init__(self, callback):
self.callback = callback
def perform_operation(self):
# 进行一些操作
result = 42
# 调用回调方法
self.callback.callback_method("arg1", "arg2")
# 使用回调方法
callback_instance = CallbackInterface()
perform_operation = PerformOperation(callback_instance)
perform_operation.perform_operation()
```
上述代码中,`CallbackInterface` 类定义了一个回调方法 `callback_method`。`PerformOperation` 类接收一个实现了 `CallbackInterface` 接口的对象作为参数,并在适当的时候调用该对象的回调方法。
无论是使用函数还是类方法来实现接口回调,关键在于正确地传递回调函数或者实现了回调接口的对象,并在适当的时候进行调用。
阅读全文
相关推荐
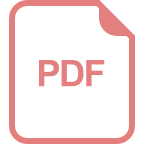
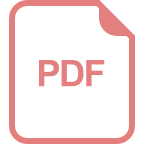

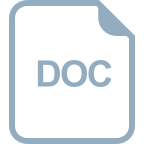


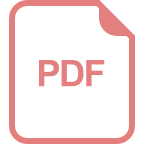
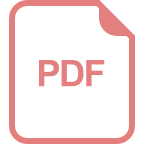
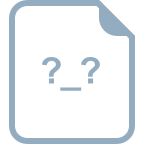
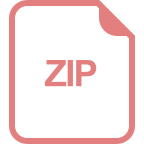
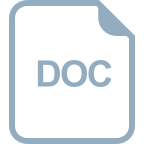
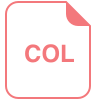



