用eclipse编写小游戏
时间: 2023-12-07 14:01:38 浏览: 313
使用Eclipse编写小游戏是一种常见的方法,Eclipse是一款功能强大的集成开发环境(IDE),适用于Java语言的开发。以下是使用Eclipse编写小游戏的简要步骤:
1. 创建一个新的Java项目:在Eclipse中,选择“File”(文件)-“New”(新建)-“Java Project"(Java项目),并为项目命名。
2. 创建游戏主类:右键单击项目文件夹,选择“New”(新建)-“Class(类)”,并命名为游戏主类,例如“Game”。
3. 在游戏主类中编写游戏逻辑:在游戏主类中定义变量、方法和游戏循环逻辑。这包括将游戏界面渲染到屏幕上,处理用户输入并更新游戏状态。
4. 创建其他必要的类:根据游戏需要,创建其他类来管理游戏中的对象,例如玩家、敌人、道具等。
5. 导入所需的资源:如果游戏需要使用图像、声音或其他资源,可以将资源文件导入到Eclipse项目中,并在代码中引用这些资源。
6. 运行和调试游戏:使用Eclipse的调试功能可以帮助检查代码的正确性,并对游戏进行调试和测试。
7. 导出可执行文件:完成游戏开发后,可以使用Eclipse将项目导出为可执行的JAR文件,供其他人使用。
使用Eclipse编写小游戏可以为游戏开发者提供良好的编码环境和调试工具。然而,游戏开发还需要掌握相关的游戏设计和编程概念,如面向对象编程、绘图技术、物理引擎等。有了这些基础,结合Eclipse的便利性,我们能够更轻松地编写出功能完善的小游戏。
相关问题
用eclipse编写java猜拳小游戏
好的,下面是用Eclipse编写Java猜拳小游戏的步骤:
1. 创建新的Java工程,命名为RockPaperScissors。
2. 在src文件夹下创建一个名为com的package,然后在com包下创建一个名为rps的package。
3. 在rps包下创建一个名为Game的Java类,这个类将包含猜拳游戏的主要逻辑。
4. 在Game类中添加以下成员变量:
```
private int playerScore;
private int computerScore;
private Scanner scanner;
```
这些变量将分别用于保存玩家和计算机的得分,并用于读取用户输入。
5. 在Game类中添加以下构造函数:
```
public Game() {
playerScore = 0;
computerScore = 0;
scanner = new Scanner(System.in);
}
```
这个构造函数将用于初始化成员变量。
6. 在Game类中添加以下方法:
```
public void run() {
System.out.println("Welcome to Rock-Paper-Scissors!");
while (true) {
System.out.println("Player Score: " + playerScore);
System.out.println("Computer Score: " + computerScore);
System.out.println("Choose your move (1 = rock, 2 = paper, 3 = scissors):");
int playerMove = scanner.nextInt();
int computerMove = (int) (Math.random() * 3) + 1;
System.out.println("Computer chose: " + computerMove);
int result = getResult(playerMove, computerMove);
if (result == 0) {
System.out.println("Tie!");
} else if (result == 1) {
System.out.println("You win!");
playerScore++;
} else {
System.out.println("You lose!");
computerScore++;
}
}
}
private int getResult(int playerMove, int computerMove) {
if (playerMove == computerMove) {
return 0;
} else if ((playerMove == 1 && computerMove == 3) ||
(playerMove == 2 && computerMove == 1) ||
(playerMove == 3 && computerMove == 2)) {
return 1;
} else {
return -1;
}
}
```
这些方法将用于运行游戏并计算结果。
7. 在Game类中添加以下main方法:
```
public static void main(String[] args) {
Game game = new Game();
game.run();
}
```
这个main方法将用于启动游戏。
8. 运行程序,开始玩猜拳游戏!
希望这个步骤能够帮助你完成用Eclipse编写Java猜拳小游戏的过程。
java用eclipse写一个小游戏
在Eclipse中编写一个小型游戏可以分为几个步骤,这里以简单的"井字游戏(Tic Tac Toe)"为例:
1. **项目设置**:
- 创建新项目:打开Eclipse,选择"File" -> "New" -> "Java Project",输入项目名称并创建。
2. **设计界面**:
- 使用Swing库:右键点击项目 -> "New" -> "JFrame",设计游戏主窗口和棋盘组件。
3. **核心逻辑**:
- 创建`Player`类,代表玩家A和B,每个都有一个字符标记(通常是X和O)。
- 实现`Board`类,包含9个格子和状态(如空、X、O),用于记录游戏进程。
4. **用户交互**:
- 在`JFrame`上添加`Board`作为面板,并处理鼠标事件,当用户点击格子时更新其状态并检查是否获胜或平局。
5. **游戏循环**:
- 主程序中添加一个`while`循环,不断交替让两个玩家操作,直到有胜者或平局。
6. **结果判定**:
- 在游戏结束后显示胜利者(如果有的话),或者提示平局。
7. **测试与调试**:
- 运行项目,通过Eclipse的模拟器或实际运行到桌面查看游戏效果,修复可能的问题。
```java
//简化示例代码
public class TicTacToe {
private Board board;
//...
public void startGame() {
while (board.getState() != Board.WIN && board.getState() != Board.DRAW) {
switch (board currentPlayer) { // 模拟交替玩家
case X:
currentPlayer = Player.O;
break;
case O:
currentPlayer = Player.X;
// 更新玩家位置...
}
updateUI();
}
showResult();
}
// 更多细节请参考完整代码
}
```
阅读全文
相关推荐
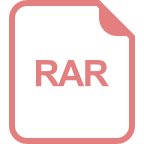
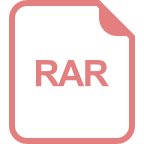
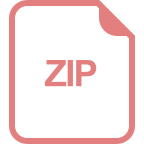













