c++string查找
时间: 2024-02-07 21:02:49 浏览: 30
C++中的string类提供了多种方法来进行查找操作。下面是一些常用的查找方法:
1. find()函数:该函数用于在字符串中查找指定的子字符串,并返回第一次出现的位置。如果未找到,则返回string::npos。
示例代码:
```cpp
std::string str = "Hello, World!";
std::size_t found = str.find("World");
if (found != std::string::npos) {
std::cout << "Substring found at position: " << found << std::endl;
} else {
std::cout << "Substring not found." << std::endl;
}
```
2. rfind()函数:与find()函数类似,但是从字符串的末尾开始查找,并返回最后一次出现的位置。
示例代码:
```cpp
std::string str = "Hello, World!";
std::size_t found = str.rfind("o");
if (found != std::string::npos) {
std::cout << "Last occurrence found at position: " << found << std::endl;
} else {
std::cout << "Substring not found." << std::endl;
}
```
3. find_first_of()函数:该函数用于在字符串中查找任意一个指定字符的第一次出现位置。
示例代码:
```cpp
std::string str = "Hello, World!";
std::size_t found = str.find_first_of("o");
if (found != std::string::npos) {
std::cout << "First occurrence of 'o' found at position: " << found << std::endl;
} else {
std::cout << "Character not found." << std::endl;
}
```
4. find_last_of()函数:与find_first_of()函数类似,但是从字符串的末尾开始查找,并返回最后一次出现的位置。
示例代码:
```cpp
std::string str = "Hello, World!";
std::size_t found = str.find_last_of("o");
if (found != std::string::npos) {
std::cout << "Last occurrence of 'o' found at position: " << found << std::endl;
} else {
std::cout << "Character not found." << std::endl;
}
```
5. find_first_not_of()函数:该函数用于在字符串中查找第一个不属于指定字符集的字符,并返回其位置。
示例代码:
```cpp
std::string str = "Hello, World!";
std::size_t found = str.find_first_not_of("Helo, Wrd!");
if (found != std::string::npos) {
std::cout << "First occurrence of character not in set found at position: " << found << std::endl;
} else {
std::cout << "No character not in set found." << std::endl;
}
```
这些是C++中string类常用的查找方法,你可以根据具体的需求选择合适的方法来进行字符串查找。
相关推荐
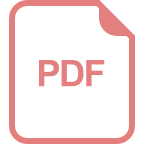














