C++编写程序实现对明文cipher的k=7,b=6的仿射加密和解密
时间: 2024-10-16 15:04:56 浏览: 27
在C++中实现仿射加密(Affine Cipher)通常涉及到两个步骤:加密和解密,其基本原理是通过将字母替换为按照特定公式计算出的新位置。对于k=7和b=6,我们可以创建一个简单的类来进行操作。这里是一个基础的示例:
```cpp
#include <iostream>
#include <string>
// 定义字符映射表,A-Z对应0-25
const char ALPHABET[] = "ABCDEFGHIJKLMNOPQRSTUVWXYZ";
class AffineCipher {
public:
// 构造函数,接收模数和偏移量作为参数
AffineCipher(int k, int b) : k_(k), b_(b) {}
// 加密函数
std::string encrypt(const std::string& plaintext) const {
std::string ciphertext;
for (char c : plaintext) {
if (isalpha(c)) { // 只处理字母字符
int index = (c - 'A') + k_ * (c - 'A') + b_;
ciphertext += ALPHABET[index % 26];
} else {
ciphertext += c; // 非字母字符保持不变
}
}
return ciphertext;
}
// 解密函数,本质上是对加密后的字符做相反的操作
std::string decrypt(const std::string& ciphertext) const {
return encrypt(ciphertext); // 因为解密是加密过程的逆运算,所以直接返回加密即可
}
private:
int k_, b_; // 模数和偏移量
bool isalpha(char c) const { return ('A' <= c && c <= 'Z'); }
};
int main() {
AffineCipher cipher(7, 6);
std::string original = "Hello, World!";
std::string encrypted = cipher.encrypt(original);
std::cout << "Encrypted text: " << encrypted << "\n";
std::string decrypted = cipher.decrypt(encrypted);
std::cout << "Decrypted text: " << decrypted << "\n";
return 0;
}
```
在这个例子中,`encrypt`方法会接收明文并应用加密规则,而`decrypt`则用于恢复原文。请注意,这个版本只适用于大写字母,如果需要处理小写字母或者其他字符,可以稍作修改。
阅读全文
相关推荐
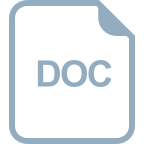
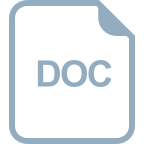
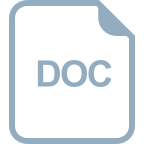
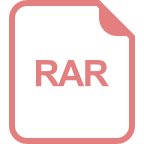
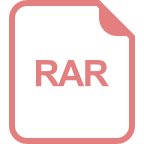
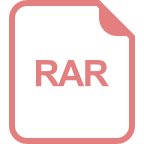
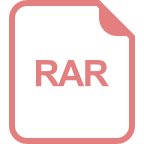
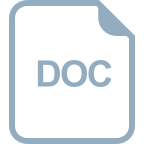
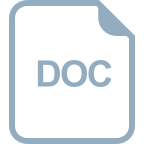




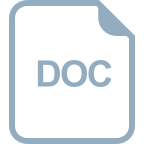
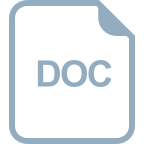
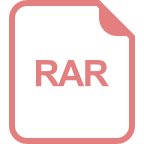
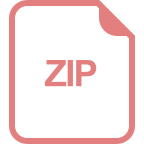
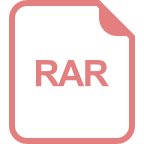
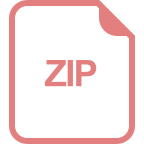