仿射密码加密解密c++
时间: 2023-10-18 20:15:51 浏览: 139
仿射密码(Affine Cipher)是一种古典密码,它是通过对明文中的每个字母进行数学变换来实现加密的。该密码的加密算法是 $E(x) = (ax + b) \mod m$,其中 $a$ 和 $b$ 是密钥参数,$m$ 是字母表长度,$x$ 是明文中的字母在字母表中的位置,$E(x)$ 是密文中的字母在字母表中的位置。
解密算法是 $D(y) = a^{-1}(y - b) \mod m$,其中 $a^{-1}$ 是 $a$ 在模 $m$ 意义下的乘法逆元,$y$ 是密文中的字母在字母表中的位置,$D(y)$ 是明文中的字母在字母表中的位置。
下面是一个简单的 C++ 实现:
```cpp
#include <iostream>
#include <string>
#include <cmath>
using namespace std;
int modInverse(int a, int m) {
a = a % m;
for (int x = 1; x < m; x++) {
if ((a * x) % m == 1) {
return x;
}
}
return -1;
}
string encrypt(string plaintext, int a, int b, int m) {
string ciphertext = "";
for (char c : plaintext) {
if (isalpha(c)) {
int x = toupper(c) - 'A';
int y = (a * x + b) % m;
ciphertext += static_cast<char>(y + 'A');
} else {
ciphertext += c;
}
}
return ciphertext;
}
string decrypt(string ciphertext, int a, int b, int m) {
string plaintext = "";
int a_inv = modInverse(a, m);
for (char c : ciphertext) {
if (isalpha(c)) {
int y = toupper(c) - 'A';
int x = (a_inv * (y - b + m)) % m;
plaintext += static_cast<char>(x + 'A');
} else {
plaintext += c;
}
}
return plaintext;
}
int main() {
string plaintext = "HELLO WORLD";
int a = 5;
int b = 8;
int m = 26;
string ciphertext = encrypt(plaintext, a, b, m);
cout << "Ciphertext: " << ciphertext << endl;
string decrypted = decrypt(ciphertext, a, b, m);
cout << "Decrypted: " << decrypted << endl;
return 0;
}
```
在上面的代码中,`modInverse` 函数是用来计算乘法逆元的,`encrypt` 函数用来加密明文,`decrypt` 函数用来解密密文。你可以将明文、密钥参数 $a$ 和 $b$、以及字母表长度 $m$ 作为函数的参数传入。在上面的例子中,我们将明文设置为 "HELLO WORLD",$a$ 设置为 5,$b$ 设置为 8,$m$ 设置为 26。
阅读全文
相关推荐
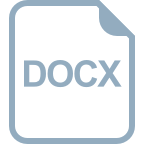


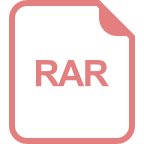
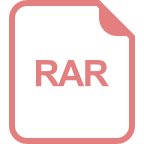
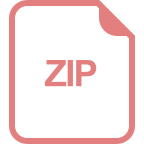
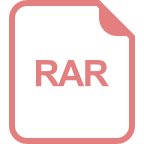
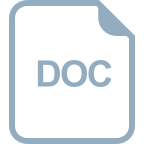
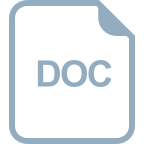
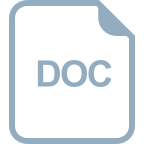




