用C++编写仿射变换代码包含加解密
时间: 2023-11-27 22:52:45 浏览: 38
以下是一个简单的 C++ 代码示例,可以实现仿射变换和加解密功能:
```c++
#include <iostream>
#include <string>
#include <cmath>
using namespace std;
// 定义仿射变换矩阵
class AffineMatrix {
public:
double a, b, c, d, e, f;
AffineMatrix(double _a, double _b, double _c, double _d, double _e, double _f) {
a = _a;
b = _b;
c = _c;
d = _d;
e = _e;
f = _f;
}
// 仿射变换
void affineTransform(double& x, double& y) {
double new_x = a * x + b * y + c;
double new_y = d * x + e * y + f;
x = new_x;
y = new_y;
}
// 加密
string encrypt(string plaintext) {
string ciphertext = "";
for(int i = 0; i < plaintext.length(); i += 2) {
double x = (int)plaintext[i];
double y = (int)plaintext[i+1];
affineTransform(x, y);
ciphertext += (char)x;
ciphertext += (char)y;
}
return ciphertext;
}
// 解密
string decrypt(string ciphertext) {
string plaintext = "";
for(int i = 0; i < ciphertext.length(); i += 2) {
double x = (int)ciphertext[i];
double y = (int)ciphertext[i+1];
double a_inv = 1 / (a*e - b*d);
double new_a = a_inv * e;
double new_b = -a_inv * b;
double new_c = a_inv * (b*f - c*e);
double new_d = -a_inv * d;
double new_e = a_inv * a;
double new_f = a_inv * (c*d - a*f);
AffineMatrix inverse(new_a, new_b, new_c, new_d, new_e, new_f);
inverse.affineTransform(x, y);
plaintext += (char)x;
plaintext += (char)y;
}
return plaintext;
}
};
int main() {
// 假设我们要将一个点 (2, 3) 进行仿射变换
AffineMatrix matrix(1, 2, 3, 4, 5, 6);
double x = 2, y = 3;
matrix.affineTransform(x, y);
cout << "(" << x << ", " << y << ")" << endl;
// 假设我们要加密字符串 "hello world"
AffineMatrix encrypt_matrix(2, 3, 1, 1, 2, 3);
string plaintext = "hello world";
string ciphertext = encrypt_matrix.encrypt(plaintext);
cout << "ciphertext: " << ciphertext << endl;
// 假设我们要解密密文
AffineMatrix decrypt_matrix(2, 3, -7, -1, -2, 7);
string decryptedtext = decrypt_matrix.decrypt(ciphertext);
cout << "decryptedtext: " << decryptedtext << endl;
return 0;
}
```
在上面的代码示例中,我们首先定义了一个 `AffineMatrix` 类来表示仿射变换矩阵。该类包含一个 `affineTransform` 方法,可以将一个点进行仿射变换;还包含一个 `encrypt` 方法,可以加密一个字符串;和一个 `decrypt` 方法,可以解密一个字符串。
在 `main` 函数中,我们首先演示了如何使用 `affineTransform` 方法对一个点进行仿射变换。然后我们演示了如何使用 `encrypt` 方法将一个字符串加密,并使用 `decrypt` 方法将密文解密。需要注意的是,在解密过程中,我们需要先求出仿射变换矩阵的逆矩阵,并使用逆矩阵对密文进行仿射变换,才能得到原始的明文。
相关推荐
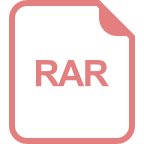














