用eclipse写一个爱心跳动代码
时间: 2024-12-11 12:37:51 浏览: 17
在Eclipse中编写一个简单的爱心(心形图案)跳动效果,通常涉及图形绘制和动画。这里以Java Swing为例,因为Eclipse支持Swing库的图形界面开发:
```java
import javax.swing.*;
import java.awt.*;
import java.awt.geom.Path2D;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
public class HeartBouncer extends JFrame {
private static final int SHAPE_SIZE = 50;
private JPanel panel;
private JLabel heartLabel;
public HeartBounce() {
setTitle("爱心跳动");
setSize(400, 400);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
initUI();
}
private void initUI() {
panel = new JPanel() {
@Override
protected void paintComponent(Graphics g) {
super.paintComponent(g);
drawHeart((int) (getHeight() / 2), SHAPE_SIZE, g);
}
};
heartLabel = new JLabel(new ImageIcon(drawHeartIcon(SHAPE_SIZE)));
add(panel, BorderLayout.CENTER);
add(heartLabel, BorderLayout.NORTH);
setLayout(new BorderLayout());
pack();
animateHeart();
}
private Image drawHeartIcon(int size) {
return Toolkit.getDefaultToolkit().createImage(
createImageBuffer(size, size)
);
}
private BufferedImage createImageBuffer(int width, int height) {
BufferedImage image = new BufferedImage(width, height, BufferedImage.TYPE_INT_ARGB);
Graphics2D g2d = image.createGraphics();
g2d.setColor(Color.RED);
g2d.setStroke(new BasicStroke(2));
drawHeart(0, 0, g2d);
g2d.dispose();
return image;
}
private void drawHeart(int x, int y, Graphics g) {
double[] controlPoints = {x + SHAPE_SIZE * 0.25, y + SHAPE_SIZE * 0.75,
x - SHAPE_SIZE * 0.25, y + SHAPE_SIZE * 0.75,
x, y + SHAPE_SIZE};
Path2D.Double path = new Path2D.Double(Path2D.WIND_EVEN_ODD, true);
path.moveTo(x, y);
path.cubicTo(controlPoints[0], controlPoints[1], controlPoints[2], controlPoints[3]);
path.lineTo(x, y);
g.draw(path);
}
private void animateHeart() {
Timer timer = new Timer(100, new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
frame.setLocation(frame.getX(), frame.getY() + SHAPE_SIZE);
if (frame.getLocationOnScreen().y >= getHeight()) {
frame.setLocation(0, 0);
animateHeart();
}
}
});
timer.start();
}
public static void main(String[] args) {
SwingUtilities.invokeLater(() -> new HeartBounce());
}
}
阅读全文
相关推荐
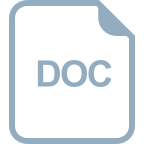
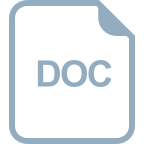
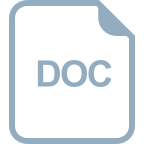
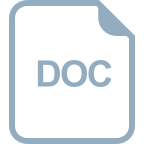
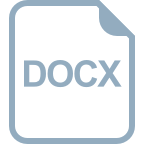
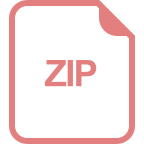
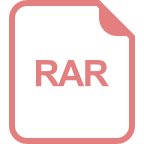
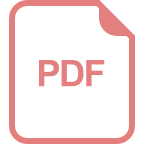
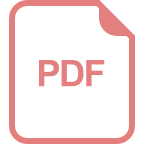
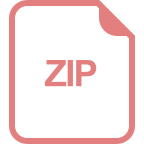
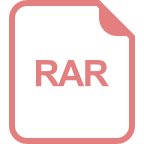
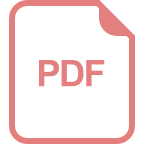
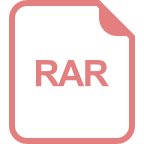
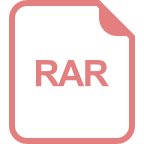
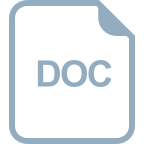