编码Python实现香农编码,要求得到编码结果、信息熵和平均码长
时间: 2024-12-19 16:23:37 浏览: 7
香农编码(Shannon Coding),又称为霍夫曼编码,是一种基于概率的无损数据压缩方法,由克劳德·香农提出。在Python中实现香农编码通常需要以下几个步骤:
1. **收集频率信息**:首先,统计输入序列中各个字符出现的概率分布。
2. **创建霍夫曼树**:根据字符及其频率构建霍夫曼树,这个过程是自底向上的,通过合并频率最小的节点直到只剩下一个根节点。
3. **生成编码规则**:从霍夫曼树的叶子节点到根节点,记录每个字符与其编码之间的映射关系。左分支代表0,右分支代表1。
4. **计算信息熵**:信息熵是指消息中的不确定性,可以用下面的公式计算:`H(X) = -∑(p(x) * log2(p(x)))`,其中`p(x)`是字符`x`出现的概率。
5. **计算平均码长**:根据编码规则,计算每个字符的平均码字长度,即其频率乘以其对应的码字长度之和除以总字符数。
以下是简单的Python实现示例(假设我们有一个包含字符'A', 'B', 'C'的小例子):
```python
import heapq
def create_frequencies(input_string):
frequencies = {char: input_string.count(char) / len(input_string) for char in set(input_string)}
return frequencies
def build_huffman_tree(frequencies):
heap = [[weight, [symbol, ""]] for symbol, weight in frequencies.items()]
heapq.heapify(heap)
while len(heap) > 1:
lo = heapq.heappop(heap)
hi = heapq.heappop(heap)
for pair in lo[1:]:
pair[1] = '0' + pair[1]
for pair in hi[1:]:
pair[1] = '1' + pair[1]
heapq.heappush(heap, [lo[0] + hi[0]] + lo[1:] + hi[1:])
return heap[0][1]
def generate_code(tree):
code_dict = {}
def _generate_codes(symbol, path=""):
if not isinstance(symbol, tuple):
code_dict[symbol] = path
else:
_generate_codes(symbol[0], path + '0')
_generate_codes(symbol[1], path + '1')
_generate_codes(tree)
return code_dict
def calculate_entropy(code_dict):
entropy = sum(-freq * math.log2(freq) for freq in code_dict.values())
return entropy
def average_code_length(code_dict, frequencies):
return sum(frequencies[char] * len(code_dict[char]) for char in code_dict)
# 示例用法
input_string = "AAABBC"
frequencies = create_frequencies(input_string)
tree = build_huffman_tree(frequencies)
code_dict = generate_code(tree)
info_entropy = calculate_entropy(code_dict)
avg_code_length = average_code_length(code_dict, frequencies)
print("编码结果:", code_dict)
print("信息熵:", info_entropy)
print("平均码长:", avg_code_length)
```
阅读全文
相关推荐
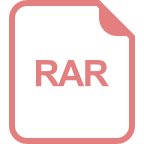
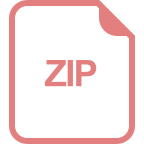
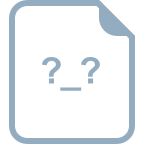





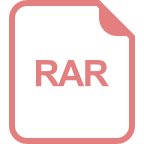

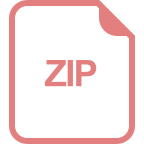
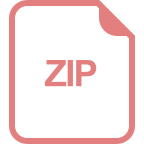
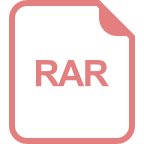
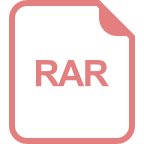
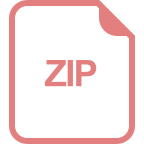
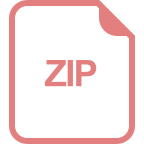
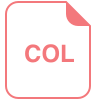
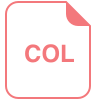
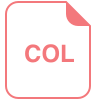