ffmpeg h264编码 流并转为rtp流 c++
时间: 2024-10-03 13:01:14 浏览: 123
FFmpeg是一个功能强大的多媒体处理工具库,它支持多种视频和音频编码格式,包括H.264编码。H264是一种高效的视频编码标准,常用于网络传输和存储。
如果你想将H264编码的视频数据流转换为 RTP (Real-Time Transport Protocol) 流,并使用 C++ 进行操作,你可以通过 FFmpeg 的 API 来完成这个过程。首先,你需要安装包含 libavcodec 和 libavformat 等FFmpeg组件的库,然后可以编写类似这样的 C++ 代码:
```cpp
#include <ffplay.h> // 对于播放器相关的API
std::string input_path = "input.mp4"; // 输入的 H.264 视频文件路径
std::string output_rtp_path = "output.rtp";
AVFormatContext* format_ctx = nullptr;
AVCodecContext* codec_ctx = nullptr;
AVPacket packet;
AVIOContext* file;
// 初始化 FFmpeg
if (avformat_open_input(&format_ctx, input_path.c_str(), NULL, NULL) != 0) {
std::cerr << "Could not open input file" << std::endl;
return -1;
}
// 寻找并打开视频编码器
AVCodec* codec = avcodec_find_encoder(AV_CODEC_ID_H264);
if (!codec) {
std::cerr << "Unsupported or missing H.264 encoder" << std::endl;
return -1;
}
codec_ctx = avcodec_alloc_context3(codec);
if (!codec_ctx) {
std::cerr << "Failed to allocate video codec context" << std::endl;
return -1;
}
// 配置编码器
/* ... 设置H264编码参数 ... */
// 打开输入文件
file = fopen(input_path.c_str(), "rb");
if (!file) {
std::cerr << "Cannot open input file for reading" << std::endl;
return -1;
}
format_ctx->pb = &file;
// 如果需要,注册RTP协议
AVOutputFormat* out_format = av_guess_format("rtp", output_rtp_path.c_str(), NULL);
if (!out_format) {
std::cerr << "Unknown output format 'rtp'" << std::endl;
return -1;
}
// 创建输出上下文
AVFormatContext* output_ctx = avformat_alloc_output_context2(NULL, NULL, out_format->name, output_rtp_path.c_str());
if (!output_ctx) {
std::cerr << "Failed to create output context" << std::endl;
return -1;
}
// 添加输出流到上下文
if (av_interleaved_write_frame(output_ctx, &packet, codec_ctx->frame)) {
std::cerr << "Failed to write frame to RTP stream" << std::endl;
return -1;
}
// 开始编码并将H264帧转换为RTP
while (av_read_frame(format_ctx, &packet) >= 0) {
if (codec->decode(packet, codec_ctx, NULL) == AVERROR(EAGAIN) || codec->decode(packet, codec_ctx, NULL) == AVERROR_EOF) {
continue; // 跳过解码错误或 EOF
}
av_packet_rescale_ts(&packet, format_ctx->time_base, output_ctx->iformat->time_base); // 调整时间戳
av_write_frame(output_ctx, &packet); // 写入 RTP 流
}
// 清理
fclose(file);
avcodec_free_context(&codec_ctx);
avformat_close_input(&format_ctx);
avformat_free_context(&output_ctx);
```
在这个示例中,你需要填写适当的H264编码参数,以及在创建输出上下文中可能需要进一步配置的RTP选项。
阅读全文
相关推荐














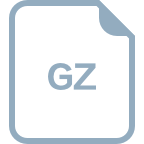
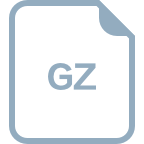