统计单词的长度 用c++
时间: 2024-09-23 20:04:53 浏览: 49
在C++中,统计单词的长度通常涉及到从输入字符串中分割单词并计算每个单词的字符数。你可以通过以下步骤完成这个任务:
1. 使用`std::string`容器来存储输入字符串,并将字符串分割成单词数组。可以使用`std::istringstream`或`std::getline`函数来逐行读取和分割。
```cpp
#include <iostream>
#include <sstream>
std::vector<std::string> splitWords(const std::string& input) {
std::istringstream iss(input);
std::vector<std::string> words;
std::string word;
while (iss >> word) {
words.push_back(word);
}
return words;
}
```
2. 创建一个辅助函数来计算单个单词的长度:
```cpp
int wordLength(const std::string& word) {
return word.length();
}
```
3. 计算所有单词的总长度并返回结果:
```cpp
int totalWordLength(const std::vector<std::string>& words) {
int sum = 0;
for (const auto& word : words) {
sum += wordLength(word);
}
return sum;
}
int main() {
std::string input = "Hello world! This is a test.";
std::vector<std::string> wordList = splitWords(input);
int totalLength = totalWordLength(wordList);
std::cout << "Total word length: " << totalLength << std::endl;
return 0;
}
```
阅读全文
相关推荐
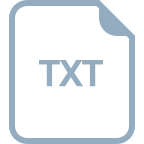
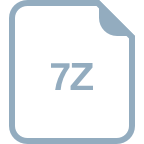
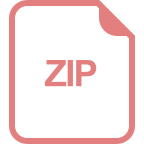



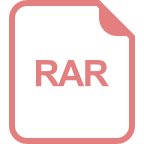
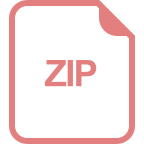
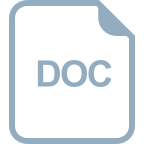
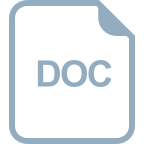
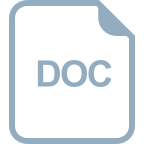
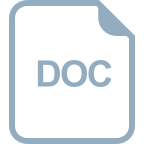






