pandas drop_duplicates返回none
时间: 2024-10-16 22:10:58 浏览: 18
当你在Pandas DataFrame上调用`drop_duplicates()`方法去除重复行时,这个方法不会直接返回None,而是直接修改原始DataFrame,删除了重复的行,并返回一个新的DataFrame(如果有的话)。如果你不想改变原数据框,你可以设置`keep='first'`(默认)或`keep='last'`来保留第一份或最后一份出现的重复项,而其他则会被删除。
例如:
```python
# 假设df是一个DataFrame
df_dedup = df.drop_duplicates()
# 或者
df_unique_first = df.drop_duplicates(keep='first')
```
如果你想查看结果是否为空,可以简单地检查返回的新DataFrame的形状(如`len(df_dedup)`),或者看是否有非空值(如`df_dedup.notnull().all().any()`)。
注意,如果你没有任何重复的行,那么`drop_duplicates()`确实不会创建新的DataFrame,但它会返回一个与原DataFrame大小相同的空DataFrame。在这种情况下,你需要确认输入的数据已经没有重复项。
相关问题
pandas drop_duplicates
The `drop_duplicates()` method in pandas is used to remove duplicate rows from a DataFrame. By default, it considers all columns and removes rows that have the same values in all columns. However, you can also specify a subset of columns to consider for identifying duplicates.
Syntax:
```
DataFrame.drop_duplicates(subset=None, keep='first', inplace=False, ignore_index=False)
```
Parameters:
- `subset`: A list of column names to consider for identifying duplicates.
- `keep`: Specifies which duplicates to keep. Possible values are `'first'` (keep the first occurrence), `'last'` (keep the last occurrence), and `False` (remove all duplicates).
- `inplace`: If `True`, the original DataFrame is modified in place and nothing is returned. If `False` (default), a new DataFrame with duplicates removed is returned.
- `ignore_index`: If `True`, the resulting DataFrame will have a new index from 0 to n-1, where n is the number of rows. If `False` (default), the resulting DataFrame will have the same index as the original DataFrame.
Example:
```
import pandas as pd
# Creating a DataFrame with duplicate rows
data = {'Name': ['John', 'John', 'Mary', 'Tom', 'Mary'],
'Age': [25, 25, 30, 35, 30],
'City': ['New York', 'New York', 'Los Angeles', 'Chicago', 'Los Angeles']}
df = pd.DataFrame(data)
# Removing duplicate rows
df.drop_duplicates(inplace=True)
print(df)
```
Output:
```
Name Age City
0 John 25 New York
2 Mary 30 Los Angeles
3 Tom 35 Chicago
4 Mary 30 Los Angeles
```
In the above example, the `drop_duplicates()` method removes the duplicate rows based on all columns and returns a new DataFrame. If `inplace=True` is used, the original DataFrame is modified directly.
pandas drop_duplicates 具体用法
`drop_duplicates()` 是 Pandas 中的一个函数,用于去除 DataFrame 或 Series 中重复的行。
具体用法如下:
```python
DataFrame.drop_duplicates(subset=None, keep='first', inplace=False, ignore_index=False)
```
其中,各参数的含义如下:
- `subset`:指定要去重的列,默认为全部列,如果指定了,则只对指定的列进行去重;
- `keep`:指定保留哪个重复的值,可选参数为 `'first'`(保留第一个)、`'last'`(保留最后一个)和 `False`(全部删除),默认为 `'first'`;
- `inplace`:是否在原 DataFrame 中进行修改,默认为 `False`,即返回一个新的 DataFrame;
- `ignore_index`:是否重新设置索引,默认为 `False`,即保留原有的索引。
例如,对如下的 DataFrame 进行去重:
```python
import pandas as pd
data = {
'name': ['Alice', 'Bob', 'Charlie', 'Bob'],
'age': [25, 30, 35, 30],
'gender': ['F', 'M', 'M', 'M']
}
df = pd.DataFrame(data)
print(df)
```
输出:
```
name age gender
0 Alice 25 F
1 Bob 30 M
2 Charlie 35 M
3 Bob 30 M
```
如果我们想要去掉重复的行,可以使用 `drop_duplicates()` 函数:
```python
df.drop_duplicates(inplace=True)
print(df)
```
输出:
```
name age gender
0 Alice 25 F
1 Bob 30 M
2 Charlie 35 M
```
可以看到,重复的行已经被去掉了。
阅读全文
相关推荐
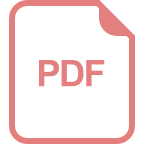
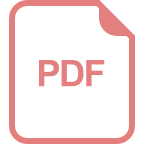
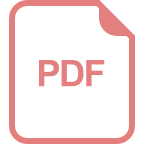










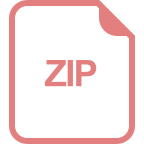