rtp发送H265例程
时间: 2023-10-22 08:05:10 浏览: 100
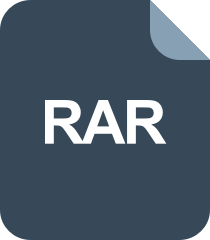
H264编解码rtp发送

以下是一个使用C++语言通过RTP发送H.265视频的简单示例:
```cpp
#include <iostream>
#include <cstdint>
#include <cstring>
#include <chrono>
#include <thread>
#include <sys/socket.h>
#include <netinet/in.h>
#include <arpa/inet.h>
// RTP头结构
struct RTPHeader {
uint8_t version:2;
uint8_t padding:1;
uint8_t extension:1;
uint8_t csrcCount:4;
uint8_t marker:1;
uint8_t payloadType:7;
uint16_t sequenceNumber;
uint32_t timestamp;
uint32_t ssrc;
};
// RTP发送器类
class RTPSender {
public:
RTPSender(const std::string& destinationIP, uint16_t destinationPort) :
destinationIP_(destinationIP), destinationPort_(destinationPort) {
// 创建UDP套接字
socket_ = socket(AF_INET, SOCK_DGRAM, 0);
// 设置远程地址信息
memset(&destinationAddr_, 0, sizeof(destinationAddr_));
destinationAddr_.sin_family = AF_INET;
destinationAddr_.sin_port = htons(destinationPort_);
inet_pton(AF_INET, destinationIP_.c_str(), &(destinationAddr_.sin_addr));
}
~RTPSender() {
// 关闭套接字
close(socket_);
}
void sendH265Packet(const uint8_t* h265Data, size_t h265Size) {
// 构建RTP头
RTPHeader header;
header.version = 2; // RTP版本
header.padding = 0; // 无填充
header.extension = 0; // 无扩展
header.csrcCount = 0; // CSRC计数为0
header.marker = 1; // 标记位,根据实际需要设置
header.payloadType = 96; // 负载类型,根据实际需要设置
header.sequenceNumber = sequenceNumber_; // 序列号,根据实际需要设置
header.timestamp = timestamp_; // 时间戳,根据实际需要设置
header.ssrc = 0; // SSRC,根据实际需要设置
// 计算RTP数据包大小
size_t rtpSize = sizeof(RTPHeader) + h265Size;
// 分配内存空间
uint8_t* rtpData = new uint8_t[rtpSize];
// 将RTP头复制到数据包
memcpy(rtpData, reinterpret_cast<uint8_t*>(&header), sizeof(RTPHeader));
// 将H.265数据复制到数据包
memcpy(rtpData + sizeof(RTPHeader), h265Data, h265Size);
// 发送RTP数据包
sendto(socket_, rtpData, rtpSize, 0,
reinterpret_cast<struct sockaddr*>(&destinationAddr_), sizeof(destinationAddr_));
// 更新序列号和时间戳
++sequenceNumber_;
timestamp_ += 90000 / 30; // 假设帧率为30fps,每帧增加1秒的时间戳
// 释放内存
delete[] rtpData;
}
private:
std::string destinationIP_;
uint16_t destinationPort_;
int socket_;
sockaddr_in destinationAddr_;
uint16_t sequenceNumber_ = 0;
uint32_t timestamp_ = 0;
};
int main() {
// RTP发送器
RTPSender rtpSender("127.0.0.1", 5004);
// H.265数据
uint8_t h265Data[] = { 0x00, 0x00, 0x00, 0x01, 0x67, 0x42, 0x00, 0x20, 0x1f, 0xff, 0xfc, 0x80, 0x00 };
// 发送H.265数据包
rtpSender.sendH265Packet(h265Data, sizeof(h265Data));
return 0;
}
```
在上述例程中,我们创建了一个 `RTPSender` 类来封装RTP发送的功能。通过指定目标IP地址和端口号,可以向指定的目标地址发送H.265数据包。
请注意,这只是一个简单的示例,你可能需要根据实际需求进行更复杂的处理和错误检测。另外,确保目标地址和端口与接收端一致,并根据实际情况设置RTP头部的字段值。
阅读全文
相关推荐

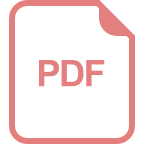
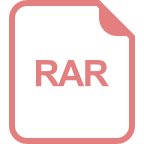
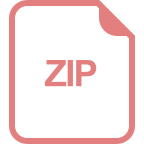
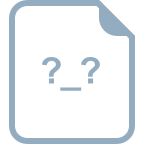
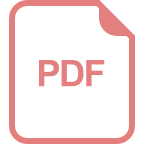
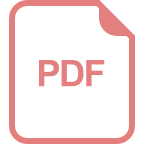
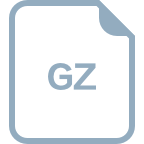



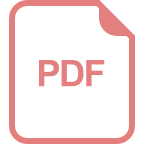
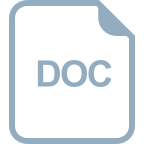