js中的try catch如何抛出错误
时间: 2024-04-27 17:15:14 浏览: 4
在JavaScript中,可以使用throw语句来抛出一个自定义的错误。当try块中的代码发生错误时,会跳转到catch块中,并执行catch块中的代码。在catch块中,可以使用throw语句来抛出一个自定义的错误,例如:
```
try {
// 可能会导致错误的代码
throw new Error('自定义错误信息');
} catch(error) {
// 错误发生时怎么处理
console.log(error.message); // 输出自定义错误信息
}
```
在上面的代码中,我们使用throw语句抛出了一个自定义的错误,并在catch块中捕获并处理了这个错误。
相关问题
js中 try catch的用法
在JavaScript中,try-catch语句用于捕获和处理可能发生的错误。它的基本语法如下:
```javascript
try {
// 可能会发生错误的代码块
} catch (error) {
// 错误处理代码块
}
```
当try代码块中的代码发生错误时,程序会立即跳转到catch代码块,并将错误信息传递给catch代码块中的error参数。你可以在catch代码块中编写适当的错误处理逻辑。
以下是一些try-catch的常见用法:
1. 捕获特定类型的错误:
```javascript
try {
// 可能会发生错误的代码块
} catch (error) {
if (error instanceof TypeError) {
// 处理类型错误
} else if (error instanceof ReferenceError) {
// 处理引用错误
} else {
// 处理其他类型的错误
}
}
```
2. 捕获多个错误:
```javascript
try {
// 可能会发生错误的代码块
} catch (error1) {
// 处理第一个错误
} catch (error2) {
// 处理第二个错误
}
```
3. 使用finally代码块:
```javascript
try {
// 可能会发生错误的代码块
} catch (error) {
// 错误处理代码块
} finally {
// 无论是否发生错误,都会执行的代码块
}
```
4. 抛出自定义错误:
```javascript
try {
throw new Error("自定义错误信息");
} catch (error) {
// 处理自定义错误
}
```
希望以上内容对你有所帮助!
try catch后返回错误信息
在使用try-catch语句后,可以通过catch块中的错误变量来获取错误信息。例如,在JavaScript中的try-catch语句中,可以使用console.log(error)来打印错误信息。在这个例子中,当try块内部的代码抛出错误时,错误信息将被传递到catch块,并通过console.log打印出来。所以,通过catch块中的错误变量,可以获取到try块内部抛出的错误信息。\[1\]
#### 引用[.reference_title]
- *1* *2* *3* *4* *5* [JavaScript try-catch 处理错误和异常指南](https://blog.csdn.net/p1967914901/article/details/127026223)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v91^insert_down28v1,239^v3^insert_chatgpt"}} ] [.reference_item]
[ .reference_list ]
相关推荐
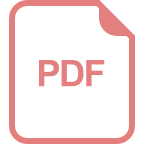
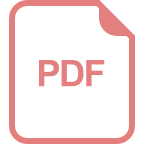
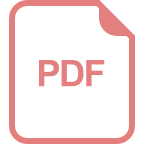
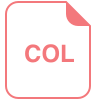
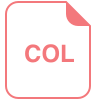
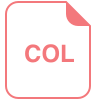
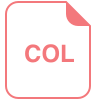
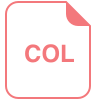







