4、统计一个段落中单词的数量(超过100个单词的段落)
时间: 2024-12-18 15:29:14 浏览: 4
统计一段文字中单词的数量,特别是在需要排除短于100个字符的段落后,可以按照以下步骤操作,这里假设你已经将段落存储在一个变量`paragraph`中:
1. 首先,你需要移除所有的标点符号和其他非字母字符。这通常通过使用Python的`string.punctuation`常量和`str.translate()`函数完成。
```python
import string
translated_paragraph = paragraph.translate(str.maketrans("", "", string.punctuation))
```
2. 接着,将文本分割成单词列表。在Python中,`split()`函数可以根据空格分隔符来做这个工作。
```python
word_list = translated_paragraph.split()
```
3. 然后,你可以检查每个单词的长度,过滤掉长度小于100的单词,并计算剩余单词的数量。
```python
filtered_words = [word for word in word_list if len(word) > 100]
word_count = len(filtered_words)
```
4. 最后,`word_count`就是大于100个字符的单词数量。
```python
print(f"段落中有 {word_count} 个超过100个单词的单词。")
```
相关问题
用re模块统计一个段落中单词的数量(超过100个单词的段落)
在Python中,你可以使用`re`模块(正则表达式库)配合`split()`函数来统计一个段落中单词的数量。首先,你需要将段落按照空格或其他标准分隔符分割成单词列表,然后计算列表长度。下面是一个简单的示例:
```python
import re
def count_words(paragraph):
# 使用正则表达式匹配单词,忽略标点符号等非字母字符
words = re.findall(r'\b\w+\b', paragraph)
# 过滤掉长度小于等于100的单词
long_words = [word for word in words if len(word) > 100]
# 返回长单词的数量
return len(long_words)
# 测试段落
paragraph_example = "这是一段超过100个单词的段落,其中包含一些非常长的单词,例如:elephant、斑马等。"
word_count = count_words(paragraph_example)
print(f"长于100个单词的单词数量:{word_count}")
阅读全文
相关推荐


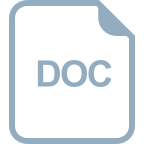








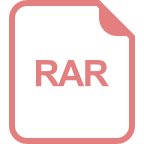

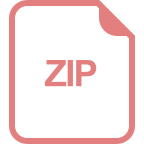


