C语言实现:给txt文件添加具体时间后缀
时间: 2024-12-21 11:22:18 浏览: 4
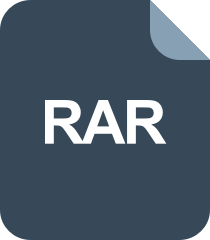
C语言遍历文件下指定后缀文件(包括子文件夹)
在C语言中,你可以使用标准库函数如`fopen`, `fclose`, `fprintf`以及一些字符串处理函数来实现给txt文件添加具体时间后缀的功能。下面是一个简单的示例,假设你想将当前时间(格式化为`yyyyMMdd_HHmmss`)追加到.txt文件名的末尾:
```c
#include <stdio.h>
#include <time.h>
// 获取当前时间并格式化为字符串
char* get_current_time() {
time_t rawtime;
struct tm * timeinfo;
char buffer[20];
time(&rawtime);
timeinfo = localtime(&rawtime);
strftime(buffer, sizeof(buffer), "%Y%m%d_%H%M%S", timeinfo);
return buffer;
}
int main() {
const char* filename = "example.txt"; // 输入的原始文件名
char timestamped_name[50]; // 结果文件名
FILE* file;
if ((file = fopen(filename, "r+")) == NULL) {
printf("无法打开文件 %s\n", filename);
return -1;
}
char* timestamp = get_current_time();
snprintf(timestamped_name, sizeof(timestamped_name), "%s_%s", filename, timestamp);
if (fclose(file)) {
perror("关闭文件失败");
return -1;
}
if ((file = fopen(timestamped_name, "a")) == NULL) {
printf("无法创建新文件 %s\n", timestamped_name);
return -1;
}
// 如果这里需要写入其他内容,可以添加写操作
fclose(file); // 关闭文件,因为已经追加了时间戳
free(timestamp); // 释放动态分配的时间戳内存
return 0;
}
```
这个程序首先尝试读取并锁定原文件,然后生成一个新的文件名,最后把原文件的指针更改为新文件,并追加数据(如果需要的话)。注意,`snprintf`用于安全地构造新的文件名,防止缓冲区溢出。
阅读全文
相关推荐
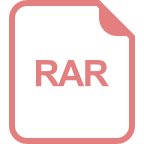
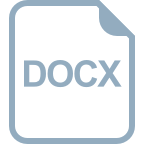
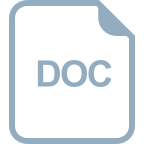
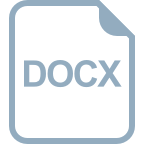
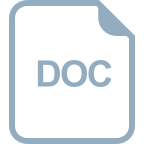
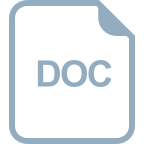
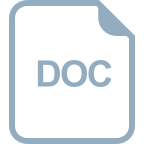
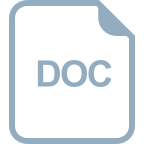
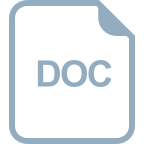
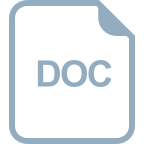
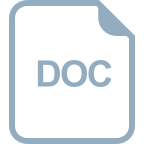
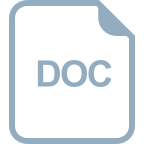
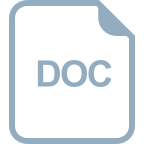
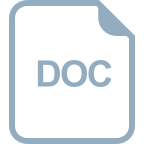
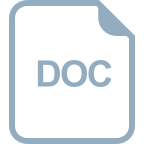
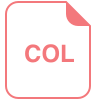

