给出一个用C++实现的大整数数据生成程序
时间: 2024-10-21 16:12:59 浏览: 15
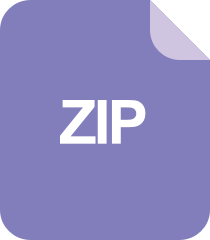
一个基于 C++ 的算法竞赛题目数据生成器.zip
在C++中,我们可以使用模板和动态内存来实现大整数数据生成程序。下面是一个简单的例子,它创建了一个`BigInt`类,用于表示任意大小的整数:
```cpp
#include <iostream>
#include <sstream>
template <typename CharT = char> // 使用char或其他类型的字符作为基础类型
class BigInt {
private:
std::vector<CharT> digits;
bool isNegative; // 标记负数
public:
BigInt() : isNegative(false) {}
BigInt(const std::string& str) { parse(str); }
BigInt(long long value) { set(value); }
void set(long long value) {
if (value < 0 && !isNegative)
isNegative = true;
for (long long i = value; abs(i) > 0; i /= 10)
digits.push_back('0' + static_cast<CharT>(i % 10));
}
void parse(const std::string& str) {
std::istringstream iss(str);
iss >> *this;
}
// ... 其他必要的操作如加法、减法等
friend std::ostream& operator<<(std::ostream& os, const BigInt& bigInt);
};
// 实现输出运算符
std::ostream& operator<<(std::ostream& os, const BigInt& bi) {
if (bi.isNegative)
os << "-";
for (const auto& digit : bi.digits)
os << digit;
return os;
}
int main() {
BigInt<int> largeNum("99999999999");
std::cout << "Large number: " << largeNum << std::endl;
return 0;
}
```
在这个示例中,我们首先定义了一个`BigInt`模板类,它可以处理不同类型的字符。然后实现了基本的构造函数(从字符串和长整型值),以及设置和解析数字的方法。为了方便输出大整数,我们还定义了`<<`运算符。
注意这只是一个基本版本,实际应用中可能需要考虑性能优化(例如更高效的加法和乘法算法)以及错误处理。
阅读全文
相关推荐
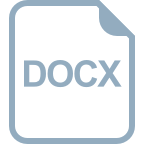
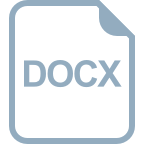
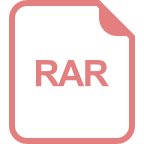
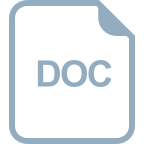
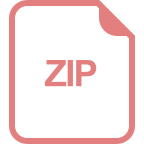
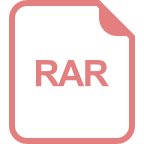
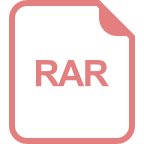
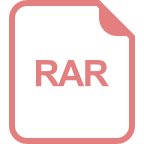
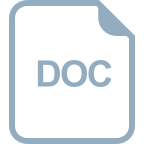
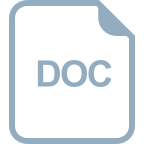
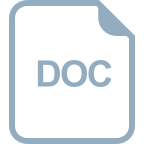
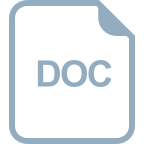
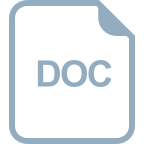
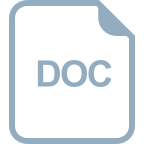



