c++读入多个不确定个数的字符串
时间: 2024-10-17 12:07:54 浏览: 29
在C++中,如果你需要从用户输入或其他数据源读取多个不确定个数的字符串,可以使用动态内存分配和循环结构来实现。这里是一个简单的示例,使用`std::getline`函数以及`std::vector<std::string>`容器来存储这些字符串:
```cpp
#include <iostream>
#include <vector>
#include <string>
int main() {
std::vector<std::string> strings;
std::string input;
// 使用while循环来持续读入直到用户输入结束标志(如空行)
while (getline(std::cin, input) && !input.empty()) {
// 检查是否需要继续读取
strings.push_back(input); // 将每条非空行添加到字符串向量中
}
// 现在strings向量包含了所有读入的字符串
for (const auto& str : strings) {
std::cout << "Read string: " << str << '\n';
}
// 为了安全起见,在不再需要字符串后释放内存
// 这里省略了实际程序中的细节,因为这通常发生在循环外部或使用智能指针
// 如果你选择手动管理内存,记得遍历并delete[]每个元素
return 0;
}
相关问题
编写一段程序从标准输入中读入多个字符串并将它们连接在一起,输出连接 成的大字符串。然后修改上述程序,用空格把输入的多个字符串分隔开来。用c++实现
以下是连接输入字符串的程序:
```c++
#include <iostream>
#include <string>
int main() {
std::string input, result;
while (std::cin >> input) { // 从标准输入中读入多个字符串
result += input; // 将它们连接起来
}
std::cout << result << std::endl; // 输出连接成的大字符串
return 0;
}
```
以下是用空格分隔输入字符串的程序:
```c++
#include <iostream>
#include <string>
int main() {
std::string input, result;
while (std::cin >> input) { // 从标准输入中读入多个字符串
result += input + " "; // 用空格把它们分隔开来并连接起来
}
std::cout << result << std::endl; // 输出连接成的大字符串
return 0;
}
```
注意,第二个程序中每个字符串的末尾都有一个空格。如果不需要这个空格,可以在输出时去掉它。
C++程序:编写一段程序从标准输入中读入多个字符串并将它们连接在一起,输出连接 成的大字符串。然后修改上述程序,用空格把输入的多个字符串分隔开来。
以下是使用 `std::string` 实现的 C++ 程序:
```cpp
#include <iostream>
#include <string>
int main() {
std::string str, result;
// 读入多个字符串并将它们连接在一起
while (std::cin >> str) {
result += str;
}
std::cout << "连接后的字符串为:" << result << std::endl;
// 用空格把输入的多个字符串分隔开来
result.clear();
std::cin.clear();
std::cin.seekg(0, std::ios::beg); // 将输入流重置到文件开头
while (std::cin >> str) {
result += str + " ";
}
std::cout << "分隔后的字符串为:" << result << std::endl;
return 0;
}
```
程序首先使用一个 `while` 循环读入多个字符串,并将它们连接在一起。连接后的大字符串存储在 `result` 变量中,最后输出。
然后程序清空 `result`,并使用 `std::cin.clear()`、`std::cin.seekg()` 将输入流重置到文件开头。再次使用 `while` 循环读入多个字符串,但是这次需要在每个字符串的末尾添加一个空格。最后输出分隔后的字符串。
阅读全文
相关推荐
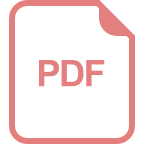
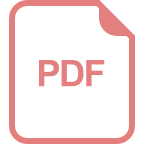
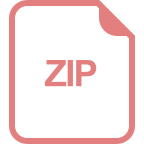













