萤火虫算法 python代码
时间: 2024-10-11 19:01:36 浏览: 35
萤火虫优化算法(Firefly Algorithm, FA)是一种模拟生物群体行为的全局搜索优化算法,灵感来源于萤火虫寻找配偶的行为。在Python中实现该算法,你可以按照以下步骤编写:
```python
import numpy as np
import random
# 定义萤火虫类
class Firefly:
def __init__(self, lower_bound, upper_bound, alpha=0.9, beta=1):
self.position = np.random.uniform(lower_bound, upper_bound)
self.intensity = np.random.uniform(0, 1)
self.alpha = alpha
self.beta = beta
# 更新位置和亮度函数
def update(self, fireflies, distance_matrix):
for other in fireflies:
if other.intensity > self.intensity:
r = distance_matrix[self.id, other.id]
new_intens = self.intensity + self.alpha * (other.intensity - self.intensity) * np.exp(-r**2 / self.beta)
self.intensity = min(max(new_intens, 0), 1) # 保持在[0,1]范围内
new_pos = self.position + self.intensity * (other.position - self.position)
self.position = np.clip(new_pos, a_min=lower_bound, a_max=upper_bound)
# 主程序
def firefly_algorithm(firefly_num, max_iter, lower_bound, upper_bound, distance_metric):
fireflies = [Firefly(lower_bound, upper_bound) for _ in range(firefly_num)]
distance_matrix = calculate_distance_matrix(fireflies, lower_bound, upper_bound, distance_metric)
for _ in range(max_iter):
for firefly in fireflies:
firefly.update(fireflies, distance_matrix)
best_firefly = sorted(fireflies, key=lambda x: x.intensity)[0]
return best_firefly.position, best_firefly.intensity
# 计算距离矩阵
def calculate_distance_matrix(fireflies, lower_bound, upper_bound, metric):
matrix = np.zeros((len(fireflies), len(fireflies)))
for i, f1 in enumerate(fireflies):
for j, f2 in enumerate(fireflies):
matrix[i, j] = metric(f1.position, f2.position, lower_bound, upper_bound)
return matrix
# 使用欧氏距离作为例子
def euclidean_distance(p1, p2, lb, ub):
return np.sqrt(np.sum((p1 - p2)**2))
max_iter = 100 # 设置最大迭代次数
firefly_num = 50 # 设置萤火虫数量
lower_bound, upper_bound = (-10, 10) # 搜索范围
best_position, best_intensity = firefly_algorithm(firefly_num, max_iter, lower_bound, upper_bound, euclidean_distance)
print("最优解:", best_position, ",亮度:", best_intensity)
阅读全文
相关推荐
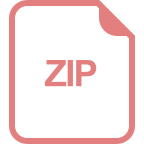
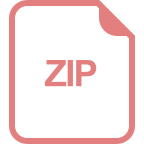
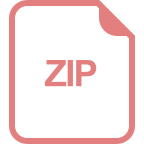
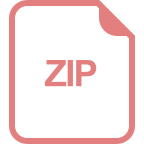
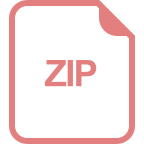
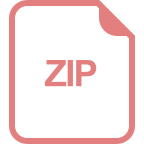
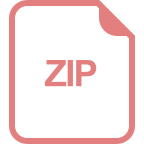
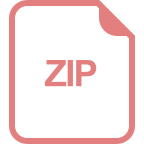
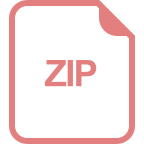
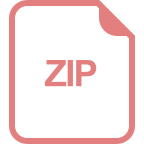