折半查找法c++从键盘输入一串数字
时间: 2024-11-22 19:46:14 浏览: 8
折半查找法(Binary Search)是一种常见的查找算法,通常用于有序数组中进行快速查找。它通过将数组从中间分开,比较中间值和目标值的大小,来确定目标值在左侧还是右侧,然后递归地在相应的一半中继续查找,直到找到目标值或者确定目标值不存在为止。
以下是使用C++实现的一个简单的折半查找算法示例:
```c++
#include <iostream>
using namespace std;
int binarySearch(int arr[], int l, int r, int x) {
if (r >= l) {
int mid = l + (r - l) / 2;
if (arr[mid] == x) {
return mid;
}
if (arr[mid] > x) {
return binarySearch(arr, l, mid - 1, x);
}
return binarySearch(arr, mid + 1, r, x);
}
return -1;
}
int main() {
int n;
cout << "请输入数组大小: ";
cin >> n;
int arr[n];
cout << "请输入 " << n << " 个数字:\n";
for (int i = 0; i < n; i++) {
cin >> arr[i];
}
int x;
cout << "请输入要查找的数字: ";
cin >> x;
int result = binarySearch(arr, 0, n - 1, x);
if (result == -1) {
cout << "未找到该数字" << endl;
} else {
cout << "该数字在数组中的索引为: " << result << endl;
}
return 0;
}
```
阅读全文
相关推荐
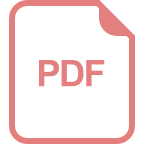
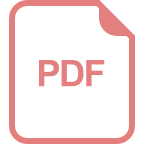
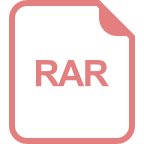
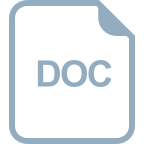
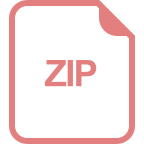
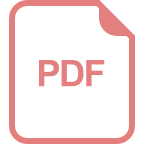
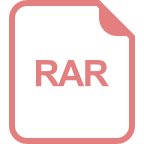
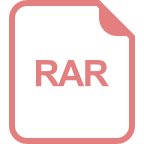
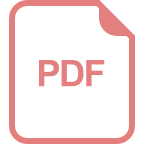
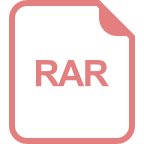
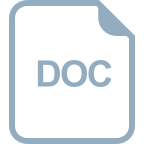