编程实现求三个数中的最大值
时间: 2024-09-26 12:04:40 浏览: 30
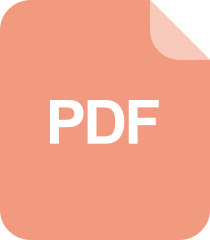
java求三个数的最大值的示例分享
在编程中,求三个数中的最大值可以使用多种方法实现,这里提供几种常见的简单示例:
1. **直接比较法**(遍历法):
```python
def find_max(a, b, c):
if a >= b and a >= c:
return a
elif b >= a and b >= c:
return b
else:
return c
# 示例
a = 5
b = 10
c = 3
max_value = find_max(a, b, c)
print("最大值是:", max_value)
```
2. **使用if-elif-else嵌套判断**:
```python
def max_of_three(a, b, c):
if a > b and a > c:
return a
elif b > a and b > c:
return b
else:
return c
# 示例
a, b, c = 7, 12, 4
maximum = max_of_three(a, b, c)
print("最大值是:", maximum)
```
3. **利用Python内置函数`max()`**:
```python
def get_max(a, b, c):
return max(a, b, c)
# 示例
numbers = [5, 10, 3]
largest_number = get_max(*numbers) # 使用星号展开列表作为函数参数
print("最大值是:", largest_number)
```
以上三种方法都是针对固定数量的三个数求最大值,如果需要处理更多个数,可以考虑使用数组或列表并利用循环结构。
阅读全文
相关推荐
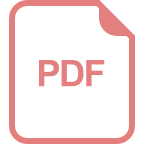
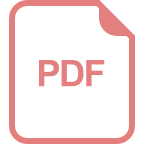
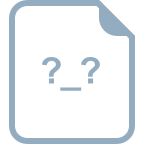
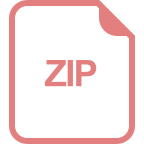
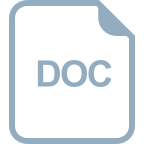
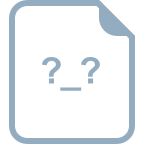
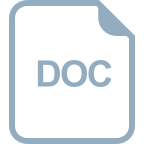
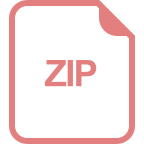
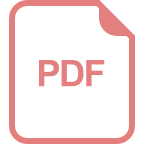
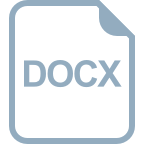
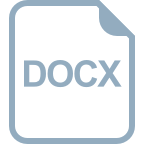
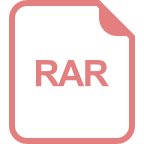
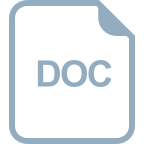
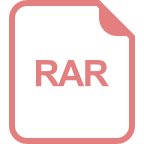
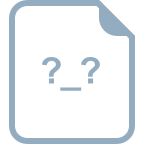
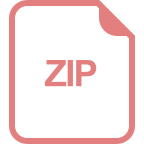
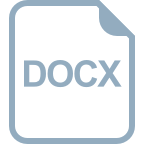