C++ 给double数值赋值 nan
时间: 2024-10-03 21:02:31 浏览: 37
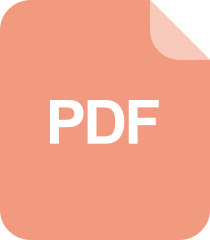
C++实现开根号的方法
在C++中,`nan`是一个特殊的浮点数,代表"非数字"(Not-a-Number),通常用于表示数学运算错误或溢出的结果。当你试图给一个`double`类型的变量赋一个超出其范围或者无法表示的值,例如除以零,结果就会变成`NaN`。
例如:
```cpp
#include <iostream>
#include <cmath>
int main() {
double num;
// 赋值一个非法的除法操作,如0除以0
num = std::numeric_limits<double>::quiet_NaN(); // 使用库函数创建NaN
// 或者直接赋值,这在某些编译器上会生成NaN
// num = 1.0 / 0.0;
if (std::isnan(num)) {
std::cout << "The value is NaN.\n";
} else {
std::cout << "The value is not NaN.\n"; // 这部分不会打印,因为num实际上是NaN
}
return 0;
}
```
阅读全文
相关推荐
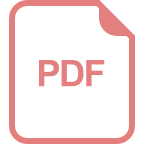
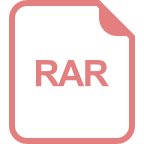
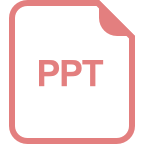
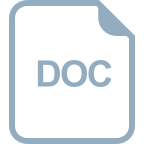
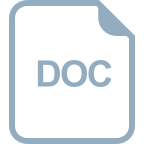
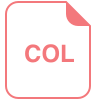
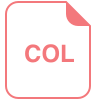




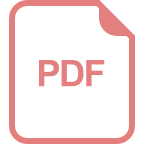
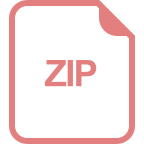
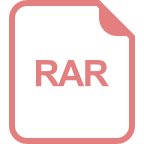
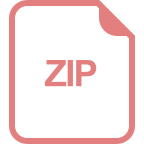
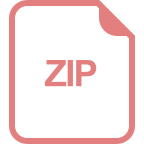
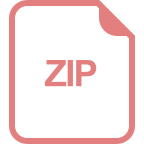
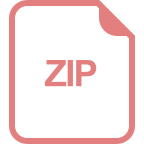