用java的数组完成以下功能,只允许使用简单函数: 6.先输入一个年份,再输入日期m月d号,输出这一天是这一年的第几天,1到365/366。注意处理闰年。 2024 4 10 101
时间: 2024-10-20 15:04:27 浏览: 30
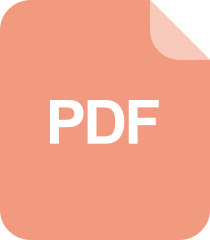
java常见的字符串操作和日期操作汇总
要完成这个功能,你可以编写一个简单的Java程序,它会接收用户输入的年份、月份和日期,然后计算给定日期是一年中的第几天。这里是一个示例程序,用于计算闰年中的天数:
```java
import java.util.Scanner;
public class DateCalculator {
public static int calculateDayInYear(int year, int month, int day) {
if (month < 1 || month > 12 || day < 1) {
throw new IllegalArgumentException("Invalid date format");
}
int daysInMonth[] = {31, 28, 31, 30, 31, 30, 31, 31, 30, 31, 30, 31}; // 默认非闰年
if (isLeapYear(year)) {
daysInMonth[1] = 29; // 如果是闰年,则二月有29天
}
int totalDays = 0;
for (int i = 0; i < month - 1; i++) {
totalDays += daysInMonth[i];
}
totalDays += day;
return totalDays;
}
private static boolean isLeapYear(int year) {
return (year % 4 == 0 && year % 100 != 0) || (year % 400 == 0);
}
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.print("Enter a year: ");
int year = scanner.nextInt();
System.out.print("Enter the month (1-12): ");
int month = scanner.nextInt();
System.out.print("Enter the day: ");
int day = scanner.nextInt();
try {
int dayOfTheYear = calculateDayInYear(year, month, day);
System.out.printf("On %d/%d/%d, it's the %dth day of the year.\n", year, month, day, dayOfTheYear);
} catch (IllegalArgumentException e) {
System.out.println(e.getMessage());
}
}
}
```
当你运行此程序并输入2024年4月10日,它将输出"101st day of the year",表示这一天是2024年的第101天。
阅读全文