string的字符串翻转c++
时间: 2023-08-16 09:10:33 浏览: 174
你可以使用C++中的标准库函数`reverse()`来翻转一个字符串。以下是一个示例代码:
```cpp
#include <iostream>
#include <algorithm> // 包含reverse函数的头文件
#include <string>
int main() {
std::string str = "Hello, World!";
std::reverse(str.begin(), str.end()); // 使用reverse函数翻转字符串
std::cout << str << std::endl; // 输出翻转后的字符串
return 0;
}
```
运行上述代码,输出将会是`!dlroW ,olleH`,即原字符串的翻转结果。
相关问题
字符串翻转函数c++
字符串翻转函数可以通过几种不同的方法来实现。下面是三种常见的方法:
第一种方法是使用algorithm库中的reverse函数。这个函数可以直接将字符串进行翻转。
```
#include <iostream>
#include <algorithm>
#include <string>
using namespace std;
int main() {
string s = "hello";
reverse(s.begin(), s.end());
cout << s << endl;
return 0;
}
```
第二种方法是使用cstring库中的strrev函数。这个函数可以将字符数组进行翻转。
```
#include <iostream>
#include <cstring>
using namespace std;
int main() {
char s[] = "hello";
strrev(s);
cout << s << endl;
return 0;
}
```
第三种方法是手动编写翻转函数。通过循环将字符串中的每个字符逆序排列。
```
#include <iostream>
using namespace std;
void Reverse(char *s, int n){
for(int i=0, j=n-1; i<j; i++, j--){
char c = s[i];
s[i] = s[j];
s[j] = c;
}
}
int main() {
char s[] = "hello";
Reverse(s, 5);
cout << s << endl;
return 0;
}
```
字符串翻转 使用c++语音
以下是使用C++语言实现字符串翻转的代码:
```cpp
#include <iostream>
#include <algorithm>
#include <string>
using namespace std;
void reverseString(string& str) {
int left = 0;
int right = str.length() - 1;
while (left < right) {
swap(str[left], str[right]);
left++;
right--;
}
}
int main() {
string str;
getline(cin, str);
reverseString(str);
cout << str << endl;
return 0;
}
```
这段代码会将输入的字符串进行翻转并输出。希望对你有帮助!如果还有其他问题,请随时提问。
阅读全文
相关推荐
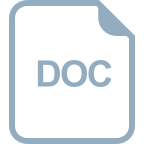
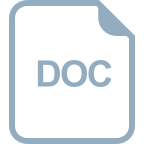
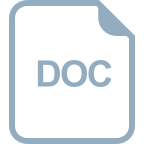
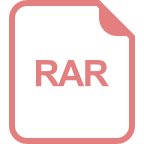
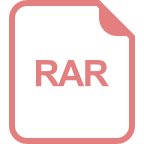











