计算的数据四舍五入为小数点后两位
时间: 2024-09-20 17:02:26 浏览: 46
在JavaScript中,你可以使用`toFixed()`方法来实现浮点数保留两位小数并四舍五入[^1]:
```javascript
let num = 3.14159;
let s_x = num.toFixed(2); // 四舍五入到小数点后两位
let pos_decimal = s_x.indexOf('.') + 1; // 找到小数点位置
// 如果没有小数点,添加一个
if (pos_decimal === 0) {
s_x += '.';
}
// 如果长度不够,用0填充
while (s_x.length < 5) { // 假设最多有三位小数,包括整数部分
s_x += '0';
}
console.log(s_x);
```
在Java中,可以使用`DecimalFormat`类或者`Math.round()`方法[^2]:
```java
import java.text.DecimalFormat;
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
double num = scanner.nextDouble();
DecimalFormat df = new DecimalFormat("#.00");
String roundedStr = df.format(num); // 使用#表示要保留的小数位数
if (!roundedStr.contains(".")) {
roundedStr += ".";
}
while (roundedStr.length() <= 5) {
roundedStr += "0";
}
System.out.println(roundedStr);
}
}
```
或者直接使用`Math.round()`:
```java
double num = 3.14159;
int roundNum = Math.round(num * 100) / 100.0; // 因为Math.round返回的是long类型,所以乘以100再除以100保证结果是double类型
String roundedStr = String.format("%.2f", roundNum); // 使用format格式化字符串,保留两位小数
System.out.println(roundedStr);
```
阅读全文
相关推荐
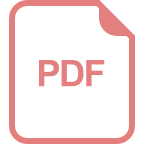
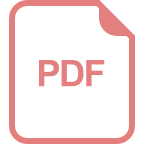
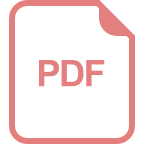




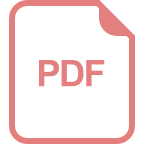
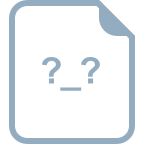
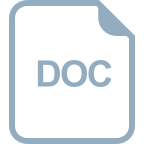
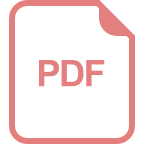
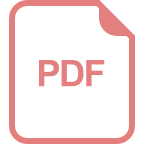
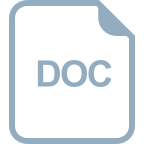





