如何在 Vue 中使用 G6 Graph 实现节点鼠标移入事件的节流处理?
时间: 2024-11-03 17:16:24 浏览: 31
在 Vue 中使用 G6 Graph 实现节点鼠标移入事件的节流处理,可以按照以下步骤操作:
首先,确保已安装了 G6 和 Vue。如果还没有,可以在项目中通过 npm 或 yarn 安装:
```bash
npm install g6 @vue/cli-plugin-g6 --save
# 或者
yarn add g6 vue-class-component --dev
```
然后,在 Vue 组件中引入 G6 和节流库(如 lodash 的 throttle 函数),并创建一个组件选项对象:
```javascript
<template>
<div ref="graphContainer" :style="{ width: '100%', height: '500px' }"></div>
</template>
<script setup>
import { useRef } from 'vue';
import G6 from '@antv/g6';
import throttle from 'lodash/throttle';
const graphRef = useRef(null);
let isHovering = false;
let timerId;
// 初始化 G6 图形
const initGraph = async () => {
const graph = new G6.Graph({
container: graphRef.value,
width: '100%',
height: '100%',
// ... 其他配置
});
// 添加节点和边等...
};
// 节流后的节点 hover 事件处理
const handleNodeHover = (e) => {
if (!isHovering) {
isHovering = true;
startHoverTimer();
}
};
const startHoverTimer = () => {
timerId = setTimeout(() => {
// 当鼠标离开节点后停止节流处理
isHovering = false;
stopHoverTimer();
// 在这里添加鼠标移入事件的回调逻辑,比如高亮节点、显示提示信息等
}, 200); // 这里是一个示例时间间隔,你可以根据需求调整
};
const stopHoverTimer = () => {
clearTimeout(timerId);
timerId = null;
};
// 渲染完成后设置 node 监听器
initGraph().then(() => {
graph.on('node:mouseover', handleNodeHover);
});
// 当组件销毁时移除监听器,防止内存泄漏
onUnmounted(() => {
graph.off('node:mouseover', handleNodeHover);
});
</script>
```
在这个例子中,我们使用了 `throttle` 来限制节点 hover 事件的触发频率,当鼠标在节点上停留一段时间后(这里是200毫秒),才会执行后续的操作(如高亮、提示)。这样就实现了节点鼠标移入事件的节流处理。
阅读全文
相关推荐
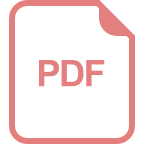
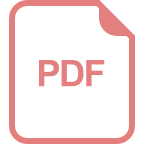
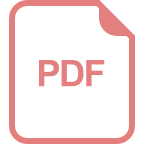
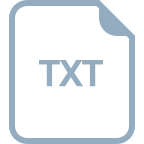
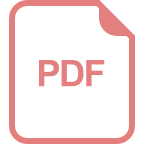
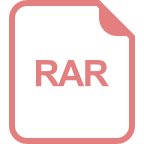
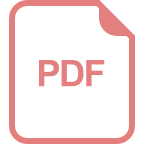
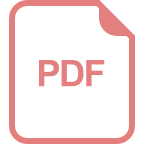
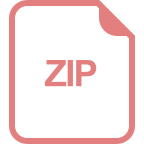
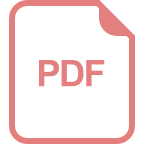
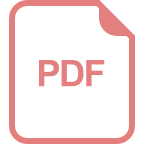
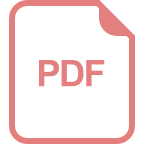
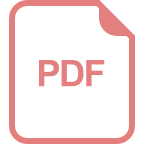
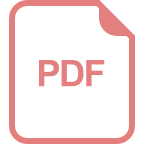
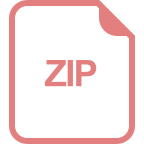
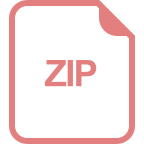
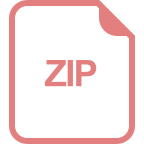