js获取当前时间年月日时分秒实例
时间: 2023-09-30 20:11:41 浏览: 110
以下是获取当前时间年月日时分秒的 JavaScript 代码示例:
```javascript
// 获取当前时间
var now = new Date();
// 获取年份
var year = now.getFullYear();
// 获取月份(注意月份从0开始,需要加1)
var month = now.getMonth() + 1;
// 获取日期
var date = now.getDate();
// 获取小时数
var hour = now.getHours();
// 获取分钟数
var minute = now.getMinutes();
// 获取秒数
var second = now.getSeconds();
// 格式化输出时间
console.log(year + '-' + month + '-' + date + ' ' + hour + ':' + minute + ':' + second);
```
输出结果可能类似于:`2021-08-01 12:34:56`。
相关问题
vue将时间戳转化为年月日时分秒格式
可以使用Vue的过滤器来将时间戳转化为年月日时分秒格式。具体实现如下:
1. 在Vue实例中定义一个过滤器:
```javascript
filters: {
formatDate: function (timestamp) {
var date = new Date(timestamp);
var year = date.getFullYear();
var month = ("0" + (date.getMonth() + 1)).slice(-2);
var day = ("0" + date.getDate()).slice(-2);
var hour = ("0" + date.getHours()).slice(-2);
var minute = ("0" + date.getMinutes()).slice(-2);
var second = ("0" + date.getSeconds()).slice(-2);
return year + "-" + month + "-" + day + " " + hour + ":" + minute + ":" + second;
}
}
```
2. 在模板中使用该过滤器:
```html
<p>{{ timestamp | formatDate }}</p>
```
其中,`timestamp`是传入过滤器的时间戳,`formatDate`是定义的过滤器名称。在模板中使用管道符号 `|` 将时间戳传入过滤器中,即可得到转化后的年月日时分秒格式。
function怎么获取年月日时分秒构成YYYY-MM-DD HH:mm:ss
在JavaScript中,你可以使用`Date`对象及其相关方法来获取当前日期和时间并格式化为"YYYY-MM-DD HH:mm:ss"的字符串形式。下面是一个示例函数,它会返回指定格式的日期时间:
```javascript
function getFormattedDateTime() {
const date = new Date(); // 获取当前日期和时间
const year = date.getFullYear(); // 年份四位数
let month = date.getMonth() + 1; // 月份,注意需要加1因为getMonth()返回0-11
const day = date.getDate(); // 月份内日子
// 格式化月份为两位数
if (month < 10) {
month = '0' + month;
}
const hours = date.getHours(); // 小时
const minutes = date.getMinutes(); // 分钟
const seconds = date.getSeconds(); // 秒数
// 如果小时、分钟或秒小于10,前面补零
if (hours < 10) {
hours = '0' + hours;
}
if (minutes < 10) {
minutes = '0' + minutes;
}
if (seconds < 10) {
seconds = '0' + seconds;
}
return `${year}-${month}-${day} ${hours}:${minutes}:${seconds}`;
}
```
现在,如果你想获取特定的日期时间,可以传入一个`Date`实例作为参数,或者直接调用这个函数获取当前时间。例如:
```javascript
const specificDate = new Date('2023-04-15T13:45:30'); // 或者不传参数,获取当前时间
console.log(getFormattedDateTime(specificDate)); // 输出格式化的日期时间
阅读全文
相关推荐
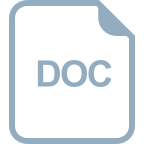
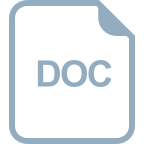
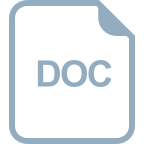
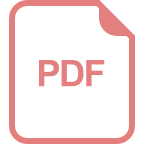
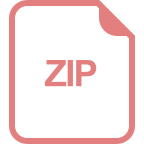
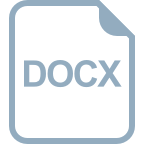
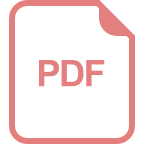
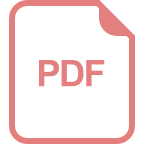
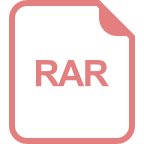
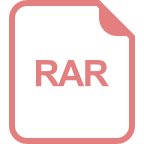
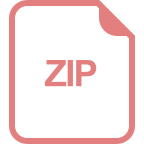
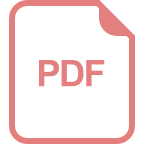
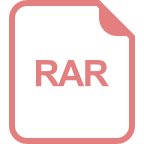
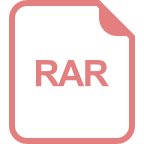
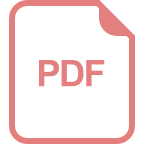
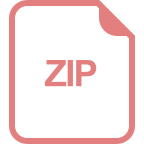
