自选图片或者通过摄像头采集的方式,分别通过harris、shi-tomasi角检测,fast、sift、orb特征点检测,将检测特征点在原图标记,将标记结果显示在GUI界面上代码
时间: 2024-02-26 12:59:13 浏览: 31
下面是通过OpenCV实现自选图片通过Harris、Shi-Tomasi角检测、FAST、SIFT、ORB特征点检测,并在GUI界面上标记的Python代码:
```python
import cv2
import tkinter as tk
from PIL import Image, ImageTk
# 图像路径
img_path = "img.jpg"
# 创建窗口
root = tk.Tk()
root.title("Feature Detection")
# 加载图像
img = cv2.imread(img_path)
# 转换图像颜色通道BGR为RGB
img = cv2.cvtColor(img, cv2.COLOR_BGR2RGB)
# 图像大小缩放
scale = 800 / img.shape[1]
img = cv2.resize(img, None, fx=scale, fy=scale)
# 创建GUI显示标签
img_label = tk.Label(root)
img_label.pack()
# Harris角检测
gray = cv2.cvtColor(img, cv2.COLOR_RGB2GRAY)
dst = cv2.cornerHarris(gray, 2, 3, 0.04)
dst_norm = cv2.normalize(dst, None, 0, 255, cv2.NORM_MINMAX, cv2.CV_8U)
dst_norm_scaled = cv2.convertScaleAbs(dst_norm)
harris_img = cv2.cvtColor(img, cv2.COLOR_RGB2BGR)
harris_img[dst_norm_scaled > 0.01 * dst_norm_scaled.max()] = [0, 0, 255]
# Shi-Tomasi角检测
gray = cv2.cvtColor(img, cv2.COLOR_RGB2GRAY)
corners = cv2.goodFeaturesToTrack(gray, 100, 0.01, 10)
corners = np.int0(corners)
shi_tomasi_img = cv2.cvtColor(img, cv2.COLOR_RGB2BGR)
for i in corners:
x, y = i.ravel()
cv2.circle(shi_tomasi_img, (x, y), 3, (0, 0, 255), -1)
# FAST特征点检测
fast = cv2.FastFeatureDetector_create()
kp = fast.detect(img, None)
fast_img = cv2.cvtColor(img, cv2.COLOR_RGB2BGR)
cv2.drawKeypoints(img, kp, fast_img, color=(0, 0, 255))
# SIFT特征点检测
sift = cv2.xfeatures2d.SIFT_create()
kp, des = sift.detectAndCompute(img, None)
sift_img = cv2.cvtColor(img, cv2.COLOR_RGB2BGR)
cv2.drawKeypoints(img, kp, sift_img, color=(0, 0, 255))
# ORB特征点检测
orb = cv2.ORB_create()
kp, des = orb.detectAndCompute(img, None)
orb_img = cv2.cvtColor(img, cv2.COLOR_RGB2BGR)
cv2.drawKeypoints(img, kp, orb_img, color=(0, 0, 255))
# 将结果拼接在一起
result_img = np.hstack((harris_img, shi_tomasi_img, fast_img, sift_img, orb_img))
# 将结果显示在GUI界面上
result_img = Image.fromarray(result_img)
result_img = ImageTk.PhotoImage(image=result_img)
img_label.config(image=result_img)
img_label.image = result_img
# 进入消息循环
root.mainloop()
```
注意:本代码中使用的图像路径为`img.jpg`,请根据实际情况进行修改。此外,本代码使用的库为OpenCV和tkinter,需要提前安装。
相关推荐
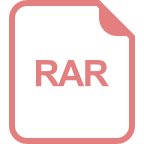
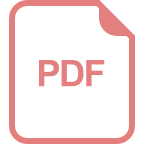
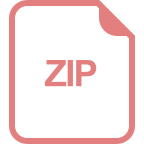









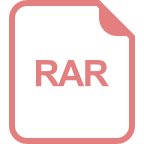
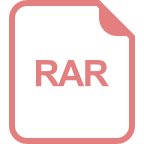
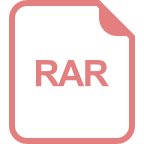
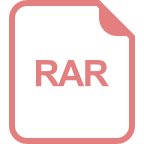
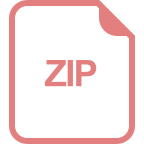