python 语义分割评价指标代码
时间: 2023-07-09 12:01:59 浏览: 154
### 回答1:
语义分割是图像处理中的一个任务,目的是将图像中的每个像素进行分类,识别出不同的物体或场景。评价指标是用来衡量模型对图像进行分割的准确程度的指标。
常用的语义分割评价指标有IoU(Intersection over Union)和mIoU(Mean Intersection over Union)。
IoU是指预测的分割结果和真实标签之间的交集面积与并集面积之比。具体计算公式为:
IoU = (预测结果与真实标签的交集面积) / (预测结果与真实标签的并集面积)
mIoU是所有图像预测结果的IoU的平均值。
以下是用Python计算语义分割评价指标的示例代码:
```python
import numpy as np
def calculate_iou(pred, target):
intersection = np.logical_and(pred, target)
union = np.logical_or(pred, target)
iou_score = np.sum(intersection) / np.sum(union)
return iou_score
def calculate_miou(preds, targets):
miou_scores = []
for pred, target in zip(preds, targets):
iou_score = calculate_iou(pred, target)
miou_scores.append(iou_score)
miou = np.mean(miou_scores)
return miou
# 假设有5个图像的预测结果和真实标签
preds = [np.array([[1, 1, 0, 0], [1, 1, 0, 0], [0, 0, 1, 1], [0, 0, 1, 1]]),
np.array([[1, 0, 0, 1], [1, 0, 0, 1], [1, 1, 0, 0], [1, 1, 0, 0]]),
np.array([[0, 0, 1, 1], [0, 0, 1, 1], [1, 0, 0, 1], [1, 0, 0, 1]]),
np.array([[1, 1, 1, 0], [1, 1, 1, 0], [0, 0, 0, 1], [0, 0, 0, 1]]),
np.array([[0, 1, 1, 0], [0, 1, 1, 0], [0, 0, 0, 1], [0, 0, 0, 1]])]
targets = [np.array([[1, 1, 0, 0], [1, 1, 0, 0], [0, 1, 1, 0], [0, 1, 1, 0]]),
np.array([[1, 0, 0, 1], [1, 0, 0, 1], [1, 1, 0, 0], [1, 1, 0, 0]]),
np.array([[0, 1, 1, 0], [0, 1, 1, 0], [1, 0, 0, 1], [1, 0, 0, 1]]),
np.array([[1, 1, 1, 0], [1, 1, 1, 0], [0, 0, 1, 1], [0, 0, 1, 1]]),
np.array([[0, 1, 1, 0], [0, 1, 1, 0], [0, 0, 1, 1], [0, 0, 1, 1]])]
miou = calculate_miou(preds, targets)
print("mIoU:", miou)
```
这段代码中,首先定义了两个函数calculate_iou和calculate_miou,用于计算IoU和mIoU。
然后创建了5个预测结果和5个真实标签的示例数据。
最后调用calculate_miou函数计算mIoU,并输出结果。
该示例只是一个简单的演示,实际应用中可能需要考虑更复杂的情况,例如处理多分类问题或处理整个数据集的评价指标。
### 回答2:
在语义分割任务中,我们常常需要评估模型的性能。以下是一些常用的语义分割评价指标及其对应的 Python 代码实现:
1. 像素准确率(Pixel Accuracy):计算预测结果中正确分类的像素数目与总像素数目的比值。
```python
def pixel_accuracy(y_true, y_pred):
total_pixels = y_true.size
correct_pixels = np.sum(y_true == y_pred)
accuracy = correct_pixels / total_pixels
return accuracy
```
2. 平均像素准确率(Mean Pixel Accuracy):计算每个类别的像素准确率的平均值。
```python
def mean_pixel_accuracy(y_true, y_pred, num_classes):
class_pixels = np.zeros(num_classes)
for c in range(num_classes):
class_pixels[c] = np.sum(np.logical_and(y_true == c, y_pred == c))
class_accuracy = class_pixels / np.sum(y_true == y_pred, axis=(0, 1))
mean_accuracy = np.mean(class_accuracy)
return mean_accuracy
```
3. 平均交并比(Mean Intersection over Union,mIOU):计算每个类别的交并比的平均值。
```python
def mean_iou(y_true, y_pred, num_classes):
class_iou = np.zeros(num_classes)
for c in range(num_classes):
intersection = np.sum(np.logical_and(y_true == c, y_pred == c))
union = np.sum(np.logical_or(y_true == c, y_pred == c))
class_iou[c] = intersection / union
mean_iou = np.mean(class_iou)
return mean_iou
```
以上是语义分割评价指标的一些示例代码。根据实际需求,也可以使用其他指标来评估模型性能。
### 回答3:
Python 语义分割评价指标代码通常用于评估语义分割模型的性能。以下是一个示例代码,用于计算语义分割模型的准确率、精确率、召回率和F1值。
```python
import numpy as np
def evaluate_semantic_segmentation(predictions, targets, num_classes):
"""
计算语义分割模型的评价指标:准确率、精确率、召回率和F1值
:param predictions: 预测的语义分割结果,形状为[H, W]
:param targets: 真实的语义分割标签,形状为[H, W]
:param num_classes: 类别数量
:return: 准确率、精确率、召回率和F1值
"""
confusion_matrix = np.zeros((num_classes, num_classes), dtype=np.int32)
for i in range(predictions.shape[0]):
for j in range(predictions.shape[1]):
predicted_class = predictions[i, j]
target_class = targets[i, j]
confusion_matrix[predicted_class, target_class] += 1
tp = np.diag(confusion_matrix)
fp = confusion_matrix.sum(axis=0) - tp
fn = confusion_matrix.sum(axis=1) - tp
accuracy = tp.sum() / confusion_matrix.sum()
precision = tp / (tp + fp)
recall = tp / (tp + fn)
f1 = (2 * precision * recall) / (precision + recall)
return accuracy, precision, recall, f1
```
这个代码中,我们首先定义了一个大小为num_classes x num_classes的混淆矩阵,用于计算预测的类别与真实的类别之间的匹配情况。然后,我们遍历预测结果和真实标签,并更新混淆矩阵。接着,我们计算真正类别(tp)、假正类别(fp)和假负类别(fn)的数量。最后,我们使用这些信息计算准确率、精确率、召回率和F1值。
相关推荐
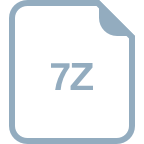
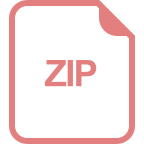














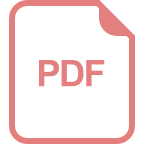