springboot mybatis插入数据
时间: 2023-08-19 19:06:19 浏览: 56
在Spring Boot中使用MyBatis插入数据,你需要进行以下几个步骤:
1. 配置数据源:在application.properties文件中配置数据库连接信息,包括驱动类、URL、用户名和密码等。[1]
2. 添加Mapper扫描:在启动类上使用@MapperScan注解,指定Mapper接口所在的包路径,以便MyBatis能够自动扫描并生成对应的实现类。[2]
3. 编写Mapper接口:创建一个Mapper接口,定义插入数据的方法。例如,在UserService接口中添加一个add方法,用于插入用户数据。[3]
4. 实现插入逻辑:在UserService的实现类中,实现add方法,调用对应的Mapper方法来插入数据。
下面是一个简单的示例代码:
```java
// UserService.java
package com.hlx.springboot.service;
import com.hlx.springboot.domain.User;
public interface UserService {
int add(User user);
}
// UserServiceImpl.java
package com.hlx.springboot.service.impl;
import com.hlx.springboot.domain.User;
import com.hlx.springboot.mapper.UserMapper;
import com.hlx.springboot.service.UserService;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
@Service
public class UserServiceImpl implements UserService {
@Autowired
private UserMapper userMapper;
@Override
public int add(User user) {
return userMapper.add(user);
}
}
// UserMapper.java
package com.hlx.springboot.mapper;
import com.hlx.springboot.domain.User;
import org.apache.ibatis.annotations.Insert;
public interface UserMapper {
@Insert("INSERT INTO user(name, age) VALUES(#{name}, #{age})")
int add(User user);
}
```
在上述示例中,我们定义了一个User实体类,包含name和age属性。在UserMapper接口中,使用@Insert注解定义了插入数据的SQL语句。在UserServiceImpl中,通过@Autowired注解将UserMapper注入,并在add方法中调用UserMapper的add方法来实现插入逻辑。
这样,当你调用UserService的add方法时,就会将数据插入到数据库中。
相关推荐
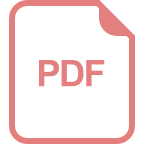














