unity udp广播 发送和接收
时间: 2023-07-29 17:13:34 浏览: 255
要在Unity中实现UDP广播的发送和接收,可以使用C#中的Socket类,以下是一个示例代码:
```csharp
using System.Net;
using System.Net.Sockets;
using System.Text;
public class UdpBroadcastSender : MonoBehaviour
{
private const int PORT = 12345;
private UdpClient udpClient;
private void Start()
{
udpClient = new UdpClient();
udpClient.EnableBroadcast = true;
udpClient.Client.SetSocketOption(SocketOptionLevel.Socket, SocketOptionName.ReuseAddress, true);
udpClient.Client.Bind(new IPEndPoint(IPAddress.Any, PORT));
}
private void OnDestroy()
{
udpClient.Close();
}
public void SendMessage(string message)
{
byte[] data = Encoding.ASCII.GetBytes(message);
udpClient.Send(data, data.Length, new IPEndPoint(IPAddress.Broadcast, PORT));
}
}
public class UdpBroadcastReceiver : MonoBehaviour
{
private const int PORT = 12345;
private UdpClient udpClient;
private IPEndPoint ipEndPoint;
private void Start()
{
udpClient = new UdpClient(PORT);
ipEndPoint = new IPEndPoint(IPAddress.Any, PORT);
udpClient.EnableBroadcast = true;
udpClient.MulticastLoopback = true;
udpClient.JoinMulticastGroup(IPAddress.Broadcast);
udpClient.BeginReceive(new AsyncCallback(OnReceive), null);
}
private void OnDestroy()
{
udpClient.Close();
}
private void OnReceive(IAsyncResult result)
{
byte[] data = udpClient.EndReceive(result, ref ipEndPoint);
string message = Encoding.ASCII.GetString(data);
Debug.Log("Received message: " + message);
udpClient.BeginReceive(new AsyncCallback(OnReceive), null);
}
}
```
在以上代码中,UdpBroadcastSender类用来发送UDP广播消息,UdpBroadcastReceiver类用来接收UDP广播消息。在UdpBroadcastReceiver类中,我们使用了异步回调的方式来接收消息,这样可以保证在接收到消息之前不会阻塞主线程。
在Unity中,你可以将上述代码分别添加到两个不同的GameObject上,并在需要发送广播消息的时候调用UdpBroadcastSender.SendMessage函数即可。同时,在接收到广播消息时,UdpBroadcastReceiver.OnReceive函数会被自动调用,你可以在这个函数中处理接收到的消息。
阅读全文
相关推荐

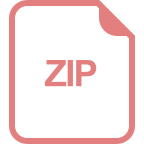



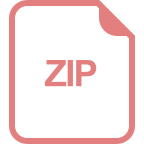
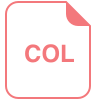


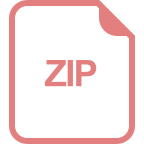
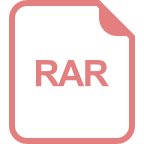
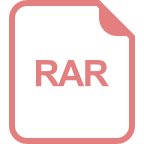
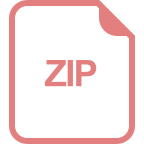
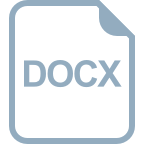
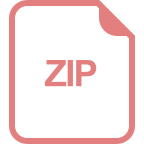