springboot发送https请求
时间: 2023-05-31 12:19:24 浏览: 88
### 回答1:
当使用Spring Boot发送HTTPS请求时,需要进行以下配置:
1. 导入证书文件
将证书文件导入到项目中,例如将证书文件放在`src/main/resources`目录下。
2. 添加Spring Security依赖
在`pom.xml`文件中添加Spring Security依赖,可以通过以下代码添加:
```
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-security</artifactId>
</dependency>
```
3. 添加HTTPS配置
在`application.properties`文件中添加HTTPS配置,例如:
```
server.port=8443
server.ssl.key-store-type=JKS
server.ssl.key-store=classpath:keystore.jks
server.ssl.key-store-password=password
```
其中,`server.port`是HTTPS服务端口号,`server.ssl.key-store-type`是证书类型,`server.ssl.key-store`是证书文件路径,`server.ssl.key-store-password`是证书密码。
4. 发送HTTPS请求
可以使用Spring的`RestTemplate`类发送HTTPS请求。以下是示例代码:
```
RestTemplate restTemplate = new RestTemplate();
HttpsURLConnection.setDefaultHostnameVerifier((hostname, sslSession) -> true);
ResponseEntity<String> response = restTemplate.exchange("https://example.com", HttpMethod.GET, null, String.class);
String result = response.getBody();
```
以上代码中,`HttpsURLConnection.setDefaultHostnameVerifier()`方法用于忽略主机名验证,因为证书中可能不包含实际使用的主机名。然后,使用`RestTemplate`发送HTTPS请求并获取响应。
### 回答2:
Spring Boot 是一个基于 Spring 框架的应用程序开发框架,它简化了应用的开发流程,提供了自动配置和容易上手的方式来构建应用。其中,在应用程序中访问 HTTPS 站点也是一项重要的需求。在这篇文章中,我们将介绍如何在 Spring Boot 应用程序中发送 HTTPS 请求。
首先,我们需要在应用程序的 pom.xml 文件中添加以下依赖项:
```xml
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-security</artifactId>
</dependency>
<dependency>
<groupId>org.apache.httpcomponents</groupId>
<artifactId>httpclient</artifactId>
</dependency>
```
这里我们添加了三个依赖项,分别用于启动 web 服务、安全认证和请求 HTTPS 站点。
接下来,我们需要配置我们的应用程序来使用 SpringSecurity 来管理 HTTPS 认证。我们可以通过在应用程序的配置类中添加以下内容来实现:
```java
@Configuration
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity http) throws Exception {
http.authorizeRequests()
.anyRequest().permitAll()
.and()
.csrf().disable();
}
}
```
这段代码禁用了 CSRF 保护,并允许所有请求。我们需要这样做是因为在 HTTPS 认证完成之前,任何的请求都将被拒绝。
接下来,我们需要创建一个 HttpClient Bean 来发送 HTTPS 请求。我们可以通过使用 HttpClientBuilder 来构建我们的 HttpClient 实例:
```java
@Configuration
public class HttpClientConfig {
@Bean
public HttpClient httpClient() throws Exception {
SSLContext sslContext = SSLContexts.custom()
.loadTrustMaterial(null, new TrustSelfSignedStrategy())
.build();
SSLConnectionSocketFactory sslConnectionSocketFactory = new SSLConnectionSocketFactory(sslContext,
NoopHostnameVerifier.INSTANCE);
return HttpClients.custom()
.setSSLSocketFactory(sslConnectionSocketFactory)
.build();
}
}
```
在这里,我们将 SSLContext 配置为信任所有证书和主机名,以允许我们访问自签名或未签名的 HTTPS 网站。
最后,我们可以在我们的 Spring Boot 应用程序中使用我们刚刚创建的 HttpClientBean 来发送 HTTPS 请求:
```java
@RestController
@RequestMapping("/")
public class MyController {
@Autowired
private HttpClient httpClient;
@GetMapping("/https")
public String getHttps() throws IOException {
HttpGet request = new HttpGet("https://example.com");
HttpResponse response = httpClient.execute(request);
String content = EntityUtils.toString(response.getEntity(), "UTF-8");
return content;
}
}
```
在这里,我们创建了一个简单的控制器,用于访问 HTTPS 站点。我们使用我们的 HttpClient Bean 来发送 HTTP 请求,然后我们解析响应并返回其内容。
总结一下,使用 SpringBoot 访问 HTTPS 站点非常简单。我们可以通过添加必要的依赖、配置 SpringSecurity 和创建 HttpClient Bean 来完成 HTTPS 认证。在我们的 SpringBoot 应用程序中,我们可以使用我们的 HttpClient Bean 来发送 HTTPS 请求,然后解析响应以获取所需的数据。
### 回答3:
SpringBoot是一种快速构建Web应用程序的框架,它已经预集成了很多常用的依赖库,使得开发者可以快速地构建出一个高效、高性能的Web应用。在实际的开发过程中,我们经常需要向第三方API发送HTTPS请求,以获取数据或与其它系统进行交互。本文将介绍如何通过SpringBoot发送HTTPS请求。
首先,在发送HTTPS请求前,我们需要导入相应的依赖。SpringBoot使用HttpClients发送HTTPS请求时,需要添加以下依赖:
```
<dependency>
<groupId>org.apache.httpcomponents</groupId>
<artifactId>httpclient</artifactId>
<version>4.5.3</version>
</dependency>
<dependency>
<groupId>org.apache.httpcomponents</groupId>
<artifactId>httpmime</artifactId>
<version>4.5.3</version>
</dependency>
```
接下来,我们需要配置SSL证书。在SpringBoot中,可以通过在application.properties文件中配置来添加SSL证书。例如,在application.properties中添加以下配置:
```
server.port=8443
server.ssl.key-store=classpath:ssl/server.p12
server.ssl.key-store-password=123456
server.ssl.keyStoreType=PKCS12
server.ssl.keyAlias=tomcat
```
其中,key-store为SSL证书的路径;key-store-password为SSL证书密码;keyStoreType为证书类型;keyAlias为证书别名。除此之外,还需要将证书加入到Java的证书库中。可以使用以下命令来添加:
```
keytool -importkeystore -destkeystore cacerts -srckeystore server.p12 -srcstoretype PKCS12 -alias tomcat
```
接下来我们就可以使用HttpClient发送HTTPS请求了。以下是一个使用HttpClient发送获取百度搜索结果的示例代码:
```
package com.example.demo.controller;
import java.io.IOException;
import java.security.KeyManagementException;
import java.security.KeyStoreException;
import java.security.NoSuchAlgorithmException;
import org.apache.http.HttpEntity;
import org.apache.http.HttpResponse;
import org.apache.http.client.HttpClient;
import org.apache.http.client.methods.HttpGet;
import org.apache.http.conn.ssl.SSLContexts;
import org.apache.http.conn.ssl.TrustSelfSignedStrategy;
import org.apache.http.impl.client.HttpClients;
import org.apache.http.util.EntityUtils;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RestController;
@RestController
public class HttpClientController {
private final String url = "https://www.baidu.com/s?wd=springboot";
@GetMapping("/baidu")
public String doGet() throws KeyManagementException, NoSuchAlgorithmException, KeyStoreException, IOException {
SSLContexts.custom().loadTrustMaterial(null, new TrustSelfSignedStrategy()).build();
HttpClient client = HttpClients.custom().setSslcontext(SSLContexts.custom().loadTrustMaterial(null, new TrustSelfSignedStrategy()).build()).build();
HttpGet httpGet = new HttpGet(url);
HttpResponse response = client.execute(httpGet);
HttpEntity entity = response.getEntity();
String result = EntityUtils.toString(entity);
return result;
}
}
```
首先,我们通过SSLContexts.custom().loadTrustMaterial()方法加载SSL证书,并将其传入到HttpClient的构造函数中。然后,通过HttpGet向百度搜索发送请求,并获取返回的数据。最后,通过EntityUtils.toString()方法将数据转换为字符串类型,并返回给前端。
以上就是使用SpringBoot发送HTTPS请求的方法。需要注意的是,在进行HTTPS请求时需要注意安全问题,对于一些重要的接口,需要对请求进行签名或者其他加密处理。
相关推荐
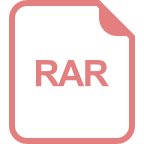
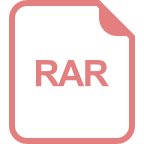














