Vector4d在C++中的用法
时间: 2024-03-27 21:41:40 浏览: 111
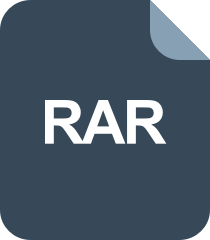
cppstlvector.rar_STL_Visual_C++_
在C++中,Vector4d通常是由程序员自己定义的一个类或结构体。以下是一个简单的Vector4d类的示例:
```c++
class Vector4d {
public:
double x, y, z, w;
Vector4d(double x = 0, double y = 0, double z = 0, double w = 0) {
this->x = x;
this->y = y;
this->z = z;
this->w = w;
}
Vector4d operator+(const Vector4d& other) const {
return Vector4d(x + other.x, y + other.y, z + other.z, w + other.w);
}
Vector4d operator-(const Vector4d& other) const {
return Vector4d(x - other.x, y - other.y, z - other.z, w - other.w);
}
Vector4d operator*(double scalar) const {
return Vector4d(x * scalar, y * scalar, z * scalar, w * scalar);
}
double dot(const Vector4d& other) const {
return x * other.x + y * other.y + z * other.z + w * other.w;
}
Vector4d cross(const Vector4d& other) const {
return Vector4d(y * other.z - z * other.y, z * other.x - x * other.z, x * other.y - y * other.x, 0);
}
double norm() const {
return sqrt(x * x + y * y + z * z + w * w);
}
void normalize() {
double n = norm();
if (n > 0) {
x /= n;
y /= n;
z /= n;
w /= n;
}
}
};
```
上述代码定义了一个四维向量类Vector4d,它包括四个成员变量x、y、z和w,以及一些常用的向量运算方法,如加减乘除、点积、叉积、求模和归一化等。程序员可以使用这个类来进行四维向量的计算和操作,例如:
```c++
Vector4d v1(1, 2, 3, 0);
Vector4d v2(4, 5, 6, 0);
Vector4d v3 = v1 + v2;
Vector4d v4 = v1 - v2;
Vector4d v5 = v1 * 2;
double dot = v1.dot(v2);
Vector4d cross = v1.cross(v2);
double norm = v1.norm();
v1.normalize();
```
以上代码演示了如何创建两个四维向量v1和v2,以及如何使用Vector4d类中的各种方法对它们进行操作和计算。
阅读全文
相关推荐
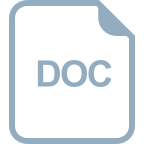
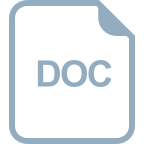
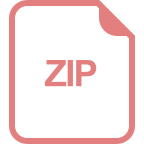
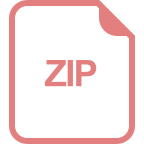
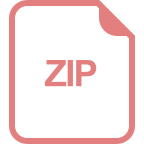
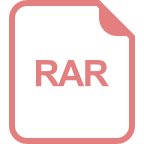
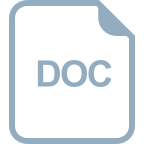
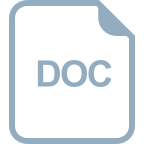
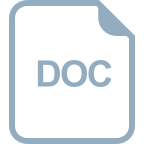
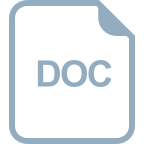
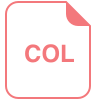
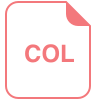
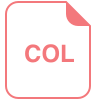
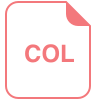
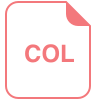
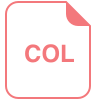

