怎么实现上传图片到数据库和读取图片展现在网页jsp+sql
时间: 2024-03-13 11:46:13 浏览: 84
下面是一个简单的示例,演示如何上传图片到数据库和将图片展示在 JSP 页面上。
1. 上传图片到数据库
在 JSP 页面上,您可以使用 `form` 标签和 `input` 标签创建一个上传表单,如下所示:
```html
<form action="upload.jsp" method="post" enctype="multipart/form-data">
<label for="file">选择文件:</label>
<input type="file" name="file" id="file">
<input type="submit" name="submit" value="上传">
</form>
```
在 `upload.jsp` 页面中,您可以使用以下代码将上传的图片保存到数据库中:
```java
// 获取上传的文件流
InputStream inputStream = request.getPart("file").getInputStream();
// 将文件流转换为字节数组
ByteArrayOutputStream outputStream = new ByteArrayOutputStream();
byte[] buffer = new byte[4096];
int bytesRead = -1;
while ((bytesRead = inputStream.read(buffer)) != -1) {
outputStream.write(buffer, 0, bytesRead);
}
byte[] bytes = outputStream.toByteArray();
// 打开数据库连接
Connection connection = null;
PreparedStatement preparedStatement = null;
try {
connection = DriverManager.getConnection("jdbc:mysql://localhost:3306/mydatabase", "root", "password");
// 创建 SQL 语句
String sql = "INSERT INTO images (name, content) VALUES (?, ?)";
preparedStatement = connection.prepareStatement(sql);
preparedStatement.setString(1, "image.jpg");
preparedStatement.setBytes(2, bytes);
// 执行 SQL 语句
preparedStatement.executeUpdate();
} catch (SQLException e) {
e.printStackTrace();
} finally {
// 关闭 preparedStatement 和数据库连接
try {
if (preparedStatement != null) {
preparedStatement.close();
}
} catch (SQLException e) {
e.printStackTrace();
}
try {
if (connection != null) {
connection.close();
}
} catch (SQLException e) {
e.printStackTrace();
}
}
```
2. 从数据库中读取图片并在 JSP 页面上展示
在 JSP 页面中,您可以使用以下代码从数据库中读取图片并将其展示在页面上:
```java
// 打开数据库连接
Connection connection = null;
PreparedStatement preparedStatement = null;
ResultSet resultSet = null;
try {
connection = DriverManager.getConnection("jdbc:mysql://localhost:3306/mydatabase", "root", "password");
// 创建 SQL 语句
String sql = "SELECT content FROM images WHERE name = ?";
preparedStatement = connection.prepareStatement(sql);
preparedStatement.setString(1, "image.jpg");
// 执行 SQL 语句
resultSet = preparedStatement.executeQuery();
if (resultSet.next()) {
// 从结果集中获取图片内容
byte[] bytes = resultSet.getBytes("content");
// 将图片内容写入到输出流中
response.setContentType("image/jpeg");
OutputStream outputStream = response.getOutputStream();
outputStream.write(bytes);
outputStream.flush();
outputStream.close();
}
} catch (SQLException e) {
e.printStackTrace();
} finally {
// 关闭 resultSet、preparedStatement 和数据库连接
try {
if (resultSet != null) {
resultSet.close();
}
} catch (SQLException e) {
e.printStackTrace();
}
try {
if (preparedStatement != null) {
preparedStatement.close();
}
} catch (SQLException e) {
e.printStackTrace();
}
try {
if (connection != null) {
connection.close();
}
} catch (SQLException e) {
e.printStackTrace();
}
}
```
在 JSP 页面上,您可以使用以下代码将数据库中的图片展示出来:
```html
<img src="showImage.jsp">
```
其中 `showImage.jsp` 页面的内容如下:
```java
// 打开数据库连接
Connection connection = null;
PreparedStatement preparedStatement = null;
ResultSet resultSet = null;
try {
connection = DriverManager.getConnection("jdbc:mysql://localhost:3306/mydatabase", "root", "password");
// 创建 SQL 语句
String sql = "SELECT content FROM images WHERE name = ?";
preparedStatement = connection.prepareStatement(sql);
preparedStatement.setString(1, "image.jpg");
// 执行 SQL 语句
resultSet = preparedStatement.executeQuery();
if (resultSet.next()) {
// 从结果集中获取图片内容
byte[] bytes = resultSet.getBytes("content");
// 将图片内容写入到输出流中
response.setContentType("image/jpeg");
OutputStream outputStream = response.getOutputStream();
outputStream.write(bytes);
outputStream.flush();
outputStream.close();
}
} catch (SQLException e) {
e.printStackTrace();
} finally {
// 关闭 resultSet、preparedStatement 和数据库连接
try {
if (resultSet != null) {
resultSet.close();
}
} catch (SQLException e) {
e.printStackTrace();
}
try {
if (preparedStatement != null) {
preparedStatement.close();
}
} catch (SQLException e) {
e.printStackTrace();
}
try {
if (connection != null) {
connection.close();
}
} catch (SQLException e) {
e.printStackTrace();
}
}
```
注意:上述代码中的数据库连接参数和 SQL 语句需要根据您的实际情况进行修改。同时,为了简化代码,这里没有处理异常、关闭资源等情况,实际使用时需要进行处理。
阅读全文
相关推荐
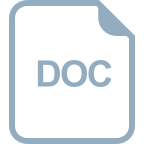
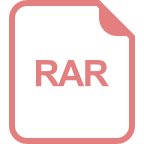
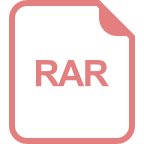
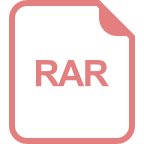
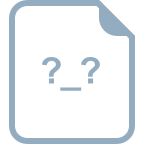
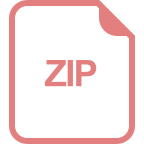
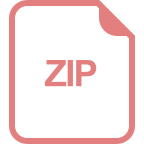
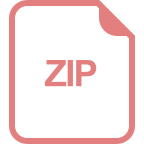
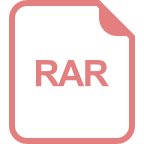
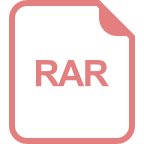
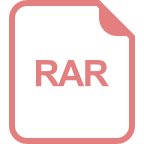
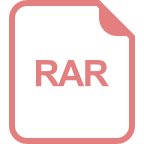
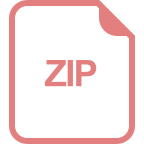
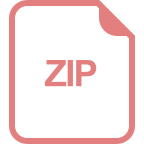
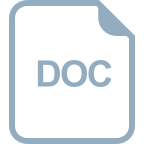
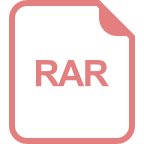
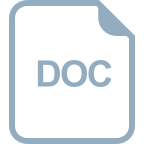