qt实现UDP组播通信
时间: 2023-08-25 20:07:40 浏览: 65
在 Qt 中实现 UDP 组播通信,需要以下步骤:
1. 创建 QUdpSocket 对象,并绑定到一个端口上:
```cpp
QUdpSocket *udpSocket = new QUdpSocket(this);
udpSocket->bind(QHostAddress::Any, port);
```
其中,port 为端口号,QHostAddress::Any 表示监听任意地址。
2. 加入组播组:
```cpp
udpSocket->joinMulticastGroup(QHostAddress(groupIP));
```
其中,groupIP 为组播组的 IP 地址。
3. 发送数据:
```cpp
QByteArray datagram = "Hello, world!";
QHostAddress address(groupIP);
udpSocket->writeDatagram(datagram.data(), datagram.size(), address, port);
```
其中,datagram 为发送的数据,address 和 port 分别为组播组的 IP 地址和端口号。
4. 接收数据:
```cpp
while (udpSocket->hasPendingDatagrams()) {
QByteArray datagram;
datagram.resize(udpSocket->pendingDatagramSize());
udpSocket->readDatagram(datagram.data(), datagram.size());
// 处理接收到的数据
}
```
在接收数据时,需要不断循环调用 hasPendingDatagrams() 方法来检测是否有数据到达,readDatagram() 方法则用于接收数据。接收到的数据保存在 datagram 变量中。
完整的示例代码:
```cpp
#include <QtNetwork>
class UdpMulticast : public QObject {
Q_OBJECT
public:
UdpMulticast(QObject *parent = nullptr)
: QObject(parent)
{
// 创建 QUdpSocket 对象
udpSocket = new QUdpSocket(this);
// 绑定到端口
udpSocket->bind(QHostAddress::Any, 5000);
// 加入组播组
udpSocket->joinMulticastGroup(QHostAddress("239.255.43.21"));
// 接收数据
connect(udpSocket, &QUdpSocket::readyRead, this, &UdpMulticast::processPendingDatagrams);
}
private slots:
void processPendingDatagrams()
{
while (udpSocket->hasPendingDatagrams()) {
QByteArray datagram;
datagram.resize(udpSocket->pendingDatagramSize());
udpSocket->readDatagram(datagram.data(), datagram.size());
qDebug() << "Received datagram:" << datagram;
}
}
private:
QUdpSocket *udpSocket;
};
```
在上面的示例中,我们创建了一个 UdpMulticast 类来实现 UDP 组播通信。在构造函数中,我们创建了一个 QUdpSocket 对象,并绑定到 5000 端口上,然后加入了组播组 239.255.43.21。
接着,我们通过 connect() 方法将 readyRead 信号连接到 processPendingDatagrams() 槽函数上,以便接收数据。在 processPendingDatagrams() 函数中,我们通过循环调用 hasPendingDatagrams() 和 readDatagram() 方法来接收数据。
相关推荐
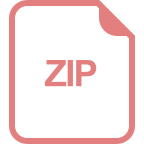














