C++实现sha1哈希的填充算法
时间: 2023-07-09 15:53:36 浏览: 186
SHA-1哈希算法中,需要对原始消息进行填充,使其长度满足512位的整数倍。填充算法步骤如下:
1. 在原始消息的末尾添加一个1,即二进制的"01"。
2. 在末尾添加一些0,直到消息的长度(以位为单位)满足以下条件:`length % 512 == 448`。
3. 在末尾添加一个64位整数,表示消息的原始长度(以位为单位),高位在前,低位在后。
C++代码示例:
```c++
#include <iostream>
#include <cstring>
#include <openssl/sha.h>
std::string sha1_padding(const std::string& str) {
// Step 1: Add "1" to the end of the message
std::string msg = str + '\x80';
// Step 2: Add "0" until length % 512 == 448
size_t length = msg.size() * 8;
size_t padding_length = (length % 512 < 448) ? (448 - length % 512) : (960 - length % 512);
msg.append(padding_length / 8, '\0');
// Step 3: Add length of the message (in bits) to the end
uint64_t length_big_endian = htobe64(length);
msg.append(reinterpret_cast<const char*>(&length_big_endian), sizeof(length_big_endian));
return msg;
}
std::string sha1(const std::string& str) {
std::string msg = sha1_padding(str);
unsigned char hash[SHA_DIGEST_LENGTH];
SHA1(reinterpret_cast<const unsigned char*>(msg.c_str()), msg.size(), hash);
std::string hash_str(reinterpret_cast<const char*>(hash), SHA_DIGEST_LENGTH);
return hash_str;
}
int main() {
std::string str = "hello world";
std::string hash = sha1(str);
std::cout << hash << std::endl;
return 0;
}
```
在上面的代码中,我们定义了两个函数:`sha1_padding`用于进行填充,`sha1`用于计算哈希值。在`sha1_padding`函数中,我们首先在字符串的末尾添加一个字符"\x80",表示二进制的"01"。然后,我们计算出填充长度,并使用`std::string::append`函数添加足够的0。最后,我们将消息的原始长度(以位为单位)转换为64位大端序整数,并将其添加到字符串的末尾。
在`sha1`函数中,我们首先使用`sha1_padding`函数对原始消息进行填充。然后,我们计算哈希值并将其转换为字符串返回。
阅读全文
相关推荐













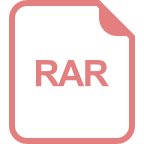

