c++ modbus RTU代码
时间: 2023-07-24 18:12:55 浏览: 465
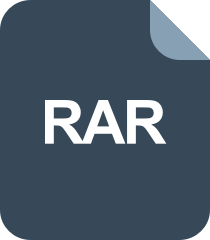
ModBusRTU.rar_MODBUS VC_ModbusRTU C_modbus_modbusRTU_modbusRTU c
下面是一个基于C++的Modbus RTU通信代码示例:
```cpp
#include <iostream>
#include <cstring>
#include <cerrno>
#include <cstdlib>
#include <cstdio>
#include <unistd.h>
#include <fcntl.h>
#include <termios.h>
using namespace std;
int open_port(const char* port) {
int fd = open(port, O_RDWR | O_NOCTTY | O_NDELAY);
if (fd == -1) {
perror("open_port: Unable to open serial port");
} else {
fcntl(fd, F_SETFL, 0);
}
return (fd);
}
int set_port(int fd, int baud_rate, int data_bits, int stop_bits, char parity) {
struct termios options;
if (tcgetattr(fd, &options) == -1) {
perror("set_port: Unable to get port settings");
return (-1);
}
cfsetispeed(&options, baud_rate);
cfsetospeed(&options, baud_rate);
options.c_cflag |= (CLOCAL | CREAD);
options.c_cflag &= ~CSIZE;
switch (data_bits) {
case 5:
options.c_cflag |= CS5;
break;
case 6:
options.c_cflag |= CS6;
break;
case 7:
options.c_cflag |= CS7;
break;
case 8:
options.c_cflag |= CS8;
break;
default:
fprintf(stderr, "Unsupported data size\n");
return (-1);
}
switch (parity) {
case 'n':
case 'N':
options.c_cflag &= ~PARENB;
options.c_iflag &= ~INPCK;
break;
case 'o':
case 'O':
options.c_cflag |= (PARODD | PARENB);
options.c_iflag |= INPCK;
break;
case 'e':
case 'E':
options.c_cflag |= PARENB;
options.c_cflag &= ~PARODD;
options.c_iflag |= INPCK;
break;
default:
fprintf(stderr, "Unsupported parity\n");
return (-1);
}
switch (stop_bits) {
case 1:
options.c_cflag &= ~CSTOPB;
break;
case 2:
options.c_cflag |= CSTOPB;
break;
default:
fprintf(stderr, "Unsupported stop bits\n");
return (-1);
}
options.c_cc[VTIME] = 0;
options.c_cc[VMIN] = 1;
tcflush(fd, TCIFLUSH);
if (tcsetattr(fd, TCSANOW, &options) == -1) {
perror("set_port: Unable to set port settings");
return (-1);
}
return (0);
}
int modbus_read_registers(int fd, unsigned char slave_addr, unsigned short start_addr, unsigned short count, unsigned short* values) {
unsigned char buf[256];
int i, len;
buf[0] = slave_addr;
buf[1] = 0x03;
buf[2] = start_addr >> 8;
buf[3] = start_addr & 0xff;
buf[4] = count >> 8;
buf[5] = count & 0xff;
len = 6;
unsigned short crc = 0xFFFF;
for (i = 0; i < len; i++) {
crc ^= buf[i];
for (int j = 0; j < 8; j++) {
if (crc & 1) {
crc >>= 1;
crc ^= 0xA001;
} else {
crc >>= 1;
}
}
}
buf[len++] = crc & 0xff;
buf[len++] = crc >> 8;
int n = write(fd, buf, len);
if (n != len) {
perror("modbus_read_registers: Unable to write to port");
return (-1);
}
usleep(10000);
len = read(fd, buf, 256);
if (len < 5) {
perror("modbus_read_registers: Error reading from port");
return (-1);
}
crc = 0xFFFF;
for (i = 0; i < len - 2; i++) {
crc ^= buf[i];
for (int j = 0; j < 8; j++) {
if (crc & 1) {
crc >>= 1;
crc ^= 0xA001;
} else {
crc >>= 1;
}
}
}
if (crc != (buf[len - 2] | (buf[len - 1] << 8))) {
perror("modbus_read_registers: CRC error");
return (-1);
}
if (buf[1] & 0x80) {
perror("modbus_read_registers: Exception response");
return (-1);
}
for (i = 0; i < count; i++) {
values[i] = buf[3 + i * 2] << 8 | buf[4 + i * 2];
}
return (0);
}
int main() {
const char* port = "/dev/ttyUSB0";
int baud_rate = B9600;
int data_bits = 8;
int stop_bits = 1;
char parity = 'N';
int fd = open_port(port);
if (fd == -1) {
return (EXIT_FAILURE);
}
if (set_port(fd, baud_rate, data_bits, stop_bits, parity) == -1) {
return (EXIT_FAILURE);
}
unsigned short values[256];
if (modbus_read_registers(fd, 1, 0, 10, values) == -1) {
return (EXIT_FAILURE);
}
for (int i = 0; i < 10; i++) {
cout << values[i] << endl;
}
close(fd);
return (EXIT_SUCCESS);
}
```
这个代码示例使用Linux系统提供的串口设备文件进行通信,并且实现了读取Modbus RTU从设备的寄存器的功能。需要注意的是,Modbus RTU通信需要进行CRC校验,这个代码示例中使用了一个CRC计算函数来实现校验。在实际使用中,你需要根据自己的具体需求进行修改。
阅读全文
相关推荐
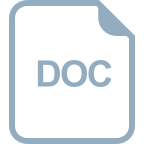
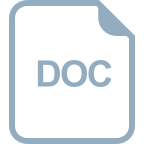



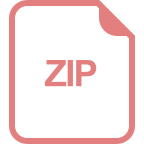
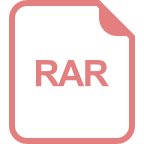
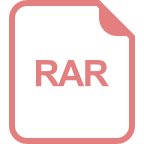




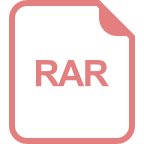
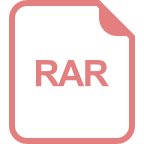
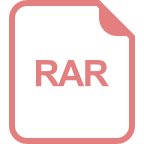
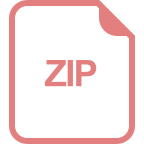
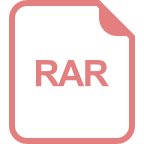