pyside制作按钮文字动画为何使用QPropertyAnimation没有效果但是setText有效果
时间: 2024-05-30 13:15:43 浏览: 172
可能是因为QPropertyAnimation是用于动画属性的值,而setText是直接设置属性的值。在按钮上使用QPropertyAnimation可能需要指定要动画的属性,例如按钮的背景颜色、边框宽度等。如果您只想动画按钮上的文字,可以尝试使用QGraphicsOpacityEffect来实现透明度动画。
相关问题
pyside使用QGraphicsOpacityEffect动画化按钮文字
下面是一个简单的示例代码,演示如何使用QGraphicsOpacityEffect动画化按钮文字:
```python
from PySide2.QtGui import *
from PySide2.QtWidgets import *
class MainWindow(QMainWindow):
def __init__(self):
super().__init__()
# 创建按钮
self.button = QPushButton("Hello World!", self)
self.button.setGeometry(50, 50, 100, 50)
# 创建QGraphicsOpacityEffect
self.opacity_effect = QGraphicsOpacityEffect(self)
self.opacity_effect.setOpacity(0.0)
self.button.setGraphicsEffect(self.opacity_effect)
# 创建动画
self.animation = QPropertyAnimation(self.opacity_effect, b"opacity")
self.animation.setDuration(1000)
self.animation.setStartValue(0.0)
self.animation.setEndValue(1.0)
# 连接按钮信号和槽函数
self.button.clicked.connect(self.animate_text)
def animate_text(self):
# 开始动画
self.animation.start()
if __name__ == "__main__":
app = QApplication([])
window = MainWindow()
window.show()
app.exec_()
```
在这个例子中,我们首先创建了一个QPushButton,并将它的Graphics Effect设置为QGraphicsOpacityEffect。我们将QGraphicsOpacityEffect的Opacity属性设置为0.0,这样按钮的文字就会变得透明。接下来,我们创建了一个QPropertyAnimation对象,用于动画化QGraphicsOpacityEffect的Opacity属性。我们将动画的起始值设置为0.0,结束值设置为1.0,这样按钮的文字就会从透明变成不透明。最后,我们连接按钮的clicked信号和animate_text槽函数,用于启动动画。当用户点击按钮时,我们将启动动画,使按钮的文字逐渐变得不透明。
pyside6 实现按钮文字消失动画
下面是一个简单的示例代码,演示了如何使用PySide6库来实现按钮文字消失动画:
```python
from PySide6.QtCore import *
from PySide6.QtGui import *
from PySide6.QtWidgets import *
class AnimatedButton(QPushButton):
def __init__(self, text='', parent=None):
super(AnimatedButton, self).__init__(text, parent)
self.text_animation = QPropertyAnimation(self, b'text')
self.text_animation.setDuration(1000)
self.text_animation.setStartValue(self.text())
self.text_animation.setEndValue('')
self.clicked.connect(self.start_animation)
def start_animation(self):
self.text_animation.start()
def setText(self, text):
super(AnimatedButton, self).setText(text)
self.update()
def text(self):
return super(AnimatedButton, self).text()
def paintEvent(self, event):
painter = QPainter(self)
painter.setRenderHint(QPainter.Antialiasing)
painter.setPen(Qt.NoPen)
painter.setBrush(self.palette().button())
rect = QRect(0, 0, self.width(), self.height())
painter.drawRoundedRect(rect, 5, 5)
font = self.font()
font.setPointSize(12)
painter.setFont(font)
painter.setPen(self.palette().text().color())
text_rect = QRect(rect)
text_rect.adjust(10, 0, -10, 0)
option = QStyleOptionButton()
option.initFrom(self)
option.text = self.text()
option.rect = text_rect
self.style().drawControl(QStyle.CE_PushButton, option, painter, self)
if __name__ == '__main__':
app = QApplication([])
button = AnimatedButton('Click me!')
button.show()
app.exec_()
```
在这个示例中,我们自定义了一个PushButton控件,名为AnimatedButton。我们使用QPropertyAnimation对象来实现按钮文字消失的动画效果。在构造函数中,我们创建了一个QPropertyAnimation对象,并将其设置为动画属性为按钮的text属性。我们还设置了动画的开始和结束值,以及其持续时间为1秒。
在按钮被点击时,我们调用start_animation()方法来启动动画。在setText()和text()方法中,我们使用QPushButton类的相应方法来实现对按钮文本的设置和获取。
在paintEvent()方法中,我们绘制了按钮的背景和边框,并使用样式绘制按钮文本。我们使用QStyleOptionButton类来设置按钮的样式选项,并使用样式绘制按钮。
阅读全文
相关推荐
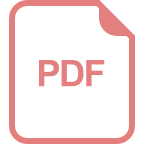
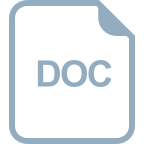
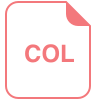

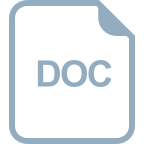









