Pyside6实现旋转动画
时间: 2024-09-26 19:13:27 浏览: 41
在PySide6中,你可以使用`QGraphicsView`和`QGraphicsScene`以及`QGraphicsProxyWidget`来创建简单的旋转动画。以下是一个基本的例子,展示了如何在一个窗口中创建一个旋转的图形:
```python
from PySide6.QtCore import Qt, QTimer
from PySide6.QtWidgets import QApplication, QMainWindow, QGraphicsView, QGraphicsScene, QGraphicsRectItem
from PySide6.QtGui import QPainter, QPixmap
class RotatingObject(QGraphicsRectItem):
def __init__(self, parent=None):
super().__init__(parent)
self.setRect(0, 0, 50, 50) # 设置初始矩形大小
self.rotation_speed = 45 # 设置旋转速度 (度/秒)
def paint(self, painter, option, widget=None):
painter.save()
painter.translate(self.pos()) # 移动到当前位置
painter.rotate(self.rotation_angle) # 根据当前角度旋转
painter.drawPixmap(0, 0, QPixmap('your_image_path')) # 画出图片
painter.restore()
@property
def rotation_angle(self):
return self.rotation_speed * QTimer.singleShotInterval() / 1000 # 计算当前的旋转角度
if __name__ == "__main__":
app = QApplication([])
window = QMainWindow()
scene = QGraphicsScene(window)
view = QGraphicsView(scene)
obj = RotatingObject() # 创建旋转对象
scene.addItem(obj)
window.setScene(scene)
window.show()
timer = QTimer()
timer.timeout.connect(lambda: obj.setRotationAngle(obj.rotation_angle))
timer.start(10) # 每10毫秒更新一次旋转角度
app.exec_()
```
在这个例子中,我们首先创建了一个继承自`QGraphicsRectItem`的`RotatingObject`类,然后定义了`paint`方法用于绘制旋转的图像。`QTimer`被用来定期调用`setRotationAngle`方法,从而改变`rotation_angle`并让图形旋转。
请确保替换`'your_image_path'`为你要使用的图片的实际路径。如果你没有图片文件,也可以用`pixmap = QPixmap(':/image.png')`(假设你的应用资源中有名为'image.png'的图片)。
阅读全文
相关推荐
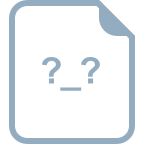
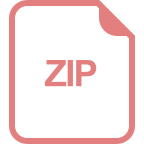
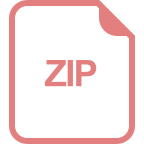

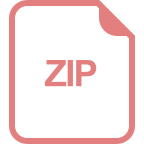
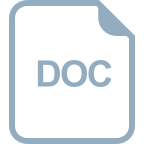
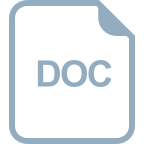
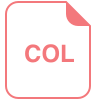


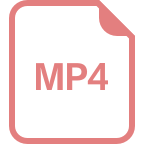
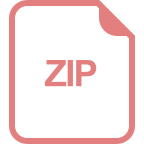
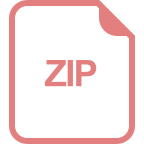
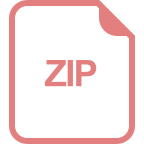
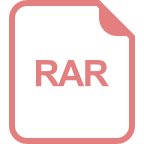
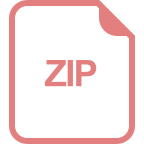
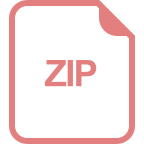
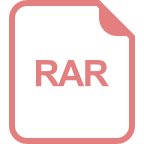