生成ifstream读取txt文件的代码
时间: 2023-12-07 09:02:23 浏览: 71
以下是生成`ifstream`读取txt文件的C++代码示例:
```cpp
#include <iostream>
#include <fstream>
#include <string>
int main() {
// 打开文件
std::ifstream file("example.txt");
// 检查文件是否打开成功
if (!file.is_open()) {
std::cerr << "Failed to open file!" << std::endl;
return 1;
}
// 读取文件内容
std::string line;
while (std::getline(file, line)) {
std::cout << line << std::endl;
}
// 关闭文件
file.close();
return 0;
}
```
在上面的代码中,我们首先创建了一个名为`file`的`ifstream`对象,并使用文件名`example.txt`初始化它。然后,我们检查文件是否成功打开,如果没有,则输出错误消息并返回1。
接下来,我们使用`std::getline`函数从文件中逐行读取内容,并将每行内容存储在名为`line`的字符串中。在读取完整个文件后,我们关闭文件并返回0。
阅读全文
相关推荐
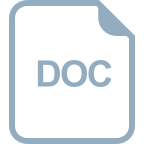
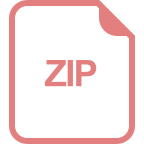
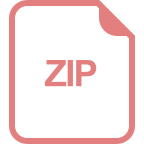
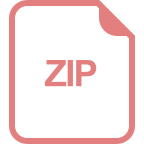
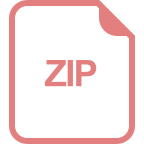
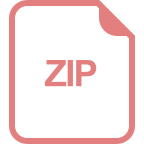
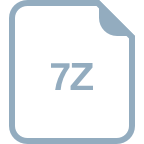
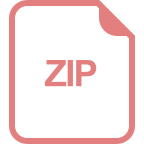
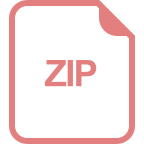
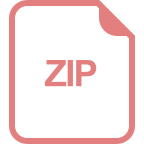
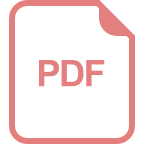
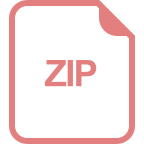
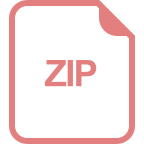
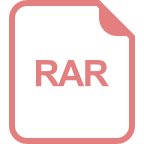
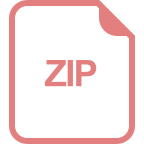