帮我用c#写一个ini文件遍历的示例
时间: 2023-11-13 11:03:51 浏览: 35
好的,下面是一个简单的示例:
```csharp
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Runtime.InteropServices;
class IniFile
{
private string filePath;
[DllImport("kernel32")]
private static extern long WritePrivateProfileString(string section,
string key, string val, string filePath);
[DllImport("kernel32")]
private static extern int GetPrivateProfileString(string section,
string key, string def, StringBuilder retVal, int size, string filePath);
public IniFile(string filePath)
{
this.filePath = filePath;
}
public void Write(string section, string key, string value)
{
WritePrivateProfileString(section, key, value, this.filePath);
}
public string Read(string section, string key)
{
StringBuilder sb = new StringBuilder(255);
GetPrivateProfileString(section, key, "", sb, 255, this.filePath);
return sb.ToString();
}
public List<string> GetSections()
{
List<string> sections = new List<string>();
byte[] buffer = new byte[2048];
int len = GetPrivateProfileString(null, null, null, buffer, 2048, this.filePath);
int j = 0;
for (int i = 0; i < len; i++)
{
if (buffer[i] == 0)
{
sections.Add(Encoding.Default.GetString(buffer, j, i - j));
j = i + 1;
}
}
return sections;
}
public Dictionary<string, string> GetKeys(string section)
{
Dictionary<string, string> keys = new Dictionary<string, string>();
StringBuilder sb = new StringBuilder(255);
int len = GetPrivateProfileString(section, null, null, sb, 255, this.filePath);
int j = 0;
for (int i = 0; i < len; i++)
{
if (sb[i] == 0)
{
string key = sb.ToString(j, i - j);
string value = Read(section, key);
keys.Add(key, value);
j = i + 1;
}
}
return keys;
}
public void RemoveKey(string section, string key)
{
Write(section, key, null);
}
public void RemoveSection(string section)
{
Write(section, null, null);
}
}
class Program
{
static void Main(string[] args)
{
IniFile ini = new IniFile("example.ini");
// 写入数据
ini.Write("Section1", "Key1", "Value1");
ini.Write("Section1", "Key2", "Value2");
ini.Write("Section2", "Key3", "Value3");
ini.Write("Section2", "Key4", "Value4");
// 读取数据
Console.WriteLine(ini.Read("Section1", "Key1"));
// 获取所有Section
List<string> sections = ini.GetSections();
foreach (string section in sections)
{
Console.WriteLine(section);
}
// 获取Section中的所有Key
Dictionary<string, string> keys = ini.GetKeys("Section1");
foreach (KeyValuePair<string, string> key in keys)
{
Console.WriteLine(key.Key + " = " + key.Value);
}
// 删除Key和Section
ini.RemoveKey("Section1", "Key1");
ini.RemoveSection("Section2");
}
}
```
这个示例演示了如何使用`IniFile`类来读取和写入INI文件,并遍历其中的数据。`IniFile`类包括了`Write`、`Read`、`GetSections`、`GetKeys`、`RemoveKey`和`RemoveSection`方法,可以方便地实现INI文件的增删改查操作。
相关推荐
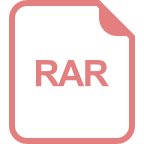
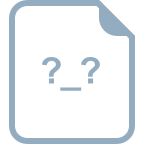
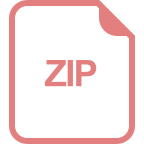














