帮我用C#写一个程序,读取本目录的DXF文件并生成图像
时间: 2024-03-05 08:54:57 浏览: 19
好的,以下是一个读取DXF文件并生成图像的C#程序示例:
```csharp
using System;
using System.Collections.Generic;
using System.Drawing;
using System.Drawing.Drawing2D;
using System.Drawing.Imaging;
using System.IO;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace DXF2Image
{
class Program
{
static void Main(string[] args)
{
// 获取本目录下所有的DXF文件
string[] files = Directory.GetFiles(".", "*.dxf");
foreach (string file in files)
{
// 读取DXF文件内容
string[] lines = File.ReadAllLines(file);
// 创建GraphicsPath对象
GraphicsPath path = new GraphicsPath();
// 遍历DXF文件中的所有LINE和LWPOLYLINE实体
foreach (string line in lines)
{
if (line.StartsWith("LINE") || line.StartsWith("LWPOLYLINE"))
{
// 获取实体的坐标点
string[] points = line.Split(new char[] { ' ' }, StringSplitOptions.RemoveEmptyEntries)
.Where(x => x.StartsWith("10") || x.StartsWith("20"))
.ToArray();
// 创建PointF数组
PointF[] pts = new PointF[points.Length / 2];
// 解析坐标点,并添加到PointF数组中
for (int i = 0; i < points.Length; i += 2)
{
float x = float.Parse(points[i + 1].Substring(2));
float y = float.Parse(points[i].Substring(2));
pts[i / 2] = new PointF(x, y);
}
// 如果是LWPOLYLINE实体,则需要连接起点和终点
if (line.StartsWith("LWPOLYLINE"))
pts[pts.Length - 1] = pts[0];
// 添加线段到GraphicsPath对象中
path.AddLines(pts);
}
}
// 创建Bitmap对象
Bitmap bmp = new Bitmap(800, 600);
// 创建Graphics对象
Graphics g = Graphics.FromImage(bmp);
// 设置Graphics对象的属性
g.SmoothingMode = SmoothingMode.AntiAlias;
g.Clear(Color.White);
// 绘制GraphicsPath对象
g.DrawPath(Pens.Black, path);
// 保存图像文件
string imgFile = Path.GetFileNameWithoutExtension(file) + ".png";
bmp.Save(imgFile, ImageFormat.Png);
// 释放资源
bmp.Dispose();
g.Dispose();
path.Dispose();
}
Console.WriteLine("完成!");
Console.ReadLine();
}
}
}
```
使用时,将DXF文件放在程序所在目录,运行程序即可生成同名的PNG图像文件。
相关推荐
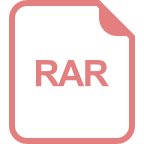














