如何使用rust实现中缀表达式转后缀表达式
时间: 2023-08-31 14:37:32 浏览: 150
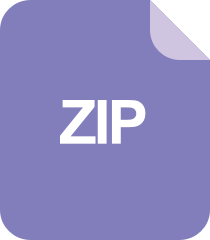
rust-regex-derivatives
### 回答1:
将中缀表达式转换为后缀表达式是一个经典的问题,在Rust中实现该算法需要遵循以下步骤:
1. 创建一个栈,用于存储运算符和括号。
2. 从左到右扫描中缀表达式。
3. 如果遇到操作数(数字),则直接将其加入到后缀表达式中。
4. 如果遇到左括号,则将其压入栈中。
5. 如果遇到右括号,则将栈顶的运算符依次弹出并加入到后缀表达式中,直到遇到左括号为止。注意,左括号只弹出不加入后缀表达式中。
6. 如果遇到运算符,则将其压入栈中。但是,在压入之前需要先将栈中优先级大于等于该运算符的运算符弹出并加入到后缀表达式中,直到遇到优先级小于该运算符的运算符为止。
7. 当扫描完整个中缀表达式后,如果栈中还有运算符,依次弹出并加入到后缀表达式中。
以下是使用Rust实现该算法的代码示例:
```rust
fn infix_to_postfix(infix: &str) -> String {
let mut stack = Vec::new();
let mut postfix = String::new();
let operators = "+-*/";
let mut iter = infix.chars().peekable();
while let Some(c) = iter.next() {
if c.is_digit(10) {
postfix.push(c);
} else if c == '(' {
stack.push(c);
} else if c == ')' {
while let Some(op) = stack.pop() {
if op == '(' {
break;
} else {
postfix.push(op);
}
}
} else if operators.contains(c) {
while let Some(op) = stack.last() {
if *op == '(' {
break;
} else if operators.find(*op).unwrap() >= operators.find(c).unwrap() {
postfix.push(stack.pop().unwrap());
} else {
break;
}
}
stack.push(c);
}
}
while let Some(op) = stack.pop() {
postfix.push(op);
}
postfix
}
```
该函数接受一个中缀表达式的字符串作为输入,返回一个后缀表达式的字符串作为输出。例如,如果输入为"(1+2)*3-4",则输出为"12+3*4-”。
### 回答2:
要使用Rust实现中缀表达式转后缀表达式,可以按照以下步骤进行:
1. 创建一个栈,用于存储运算符。
2. 遍历中缀表达式的每个字符。
3. 如果遇到操作数(数字),将其输出。
4. 如果遇到左括号"(",将其压入栈中。
5. 如果遇到右括号")",则将栈中的运算符依次弹出并输出,直到遇到左括号为止。
6. 对于遇到的运算符,如果栈为空,则将其压入栈中。
7. 如果栈不为空,比较栈顶运算符的优先级与当前运算符的优先级:
- 如果当前运算符的优先级小于或等于栈顶运算符的优先级,则将栈顶运算符弹出并输出。
- 否则,将当前运算符压入栈中。
8. 遍历完所有字符后,将栈中的剩余运算符依次弹出并输出。
这样,通过按照上述步骤处理每个字符,就可以将中缀表达式转换为后缀表达式。
以下是一个用Rust实现中缀表达式转后缀表达式的示例代码:
```rust
fn infix_to_postfix(infix_expr: &str) -> String {
let mut stack: Vec<char> = Vec::new();
let mut postfix_expr = String::new();
for c in infix_expr.chars() {
match c {
'0'..='9' => postfix_expr.push(c),
'(' => stack.push(c),
')' => {
while let Some(top) = stack.pop() {
if top == '(' {
break;
}
postfix_expr.push(top);
}
}
'+' | '-' => {
while let Some(top) = stack.last() {
if *top == '(' {
break;
}
postfix_expr.push(stack.pop().unwrap());
}
stack.push(c);
}
'*' | '/' | '%' => {
while let Some(top) = stack.last() {
if *top == '(' || *top == '+' || *top == '-' {
break;
}
postfix_expr.push(stack.pop().unwrap());
}
stack.push(c);
}
_ => {} // ignore other characters
}
}
while let Some(op) = stack.pop() {
postfix_expr.push(op);
}
postfix_expr
}
fn main() {
let infix_expr = "2+3*4";
let postfix_expr = infix_to_postfix(infix_expr);
println!("Postfix Expression: {}", postfix_expr); // Output: "234*+"
}
```
在上述示例中,我们将操作数直接输出到后缀表达式字符串中,而运算符则通过栈来处理优先级。最后输出的后缀表达式为"234*+"。
阅读全文
相关推荐
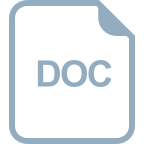
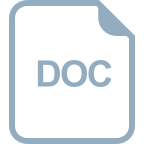
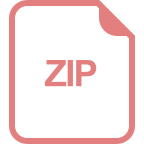
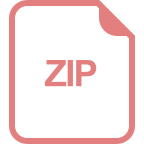
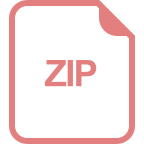
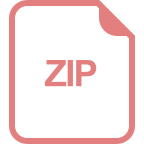
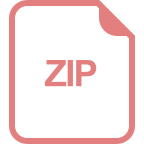
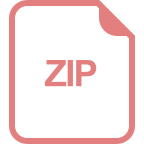
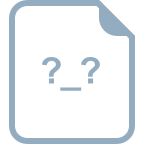
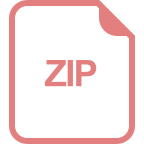
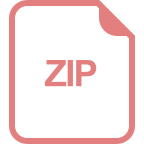
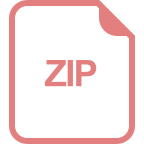
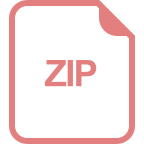
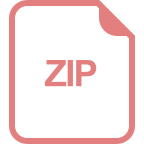
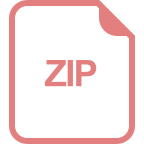


