用tcl语言写一段代码,内容是将boundary为{{0 0}{1000 1000} }的区域分割成5x5的若干方块,并输出每个方块的boundary
时间: 2023-09-04 16:03:54 浏览: 104
### 回答1:
答:set x0 0
set y0 0
set x1 1000
set y1 1000
set stepX [expr ($x1 - $x0) / 5]
set stepY [expr ($y1 - $y0) / 5]for {set i 0} {$i < 5} {incr i} {
for {set j 0} {$j < 5} {incr j} {
set x_min [expr $x0 + $i * $stepX]
set y_min [expr $y0 + $j * $stepY]
set x_max [expr $x0 + ($i + 1) * $stepX]
set y_max [expr $y0 + ($j + 1) * $stepY]
puts "boundary:{{$x_min $y_min}{$x_max $y_max}}"
}
}
### 回答2:
使用Tcl语言可以通过循环和计算来实现将指定区域分割成5x5的若干方块,并输出每个方块的boundary。以下是实现这个功能的代码示例:
```tcl
set startX 0
set startY 0
set endX 1000
set endY 1000
set squareWidth [expr {($endX - $startX) / 5}]
set squareHeight [expr {($endY - $startY) / 5}]
for {set i 0} {$i < 5} {incr i} {
for {set j 0} {$j < 5} {incr j} {
set squareStartX [expr {$startX + $i * $squareWidth}]
set squareStartY [expr {$startY + $j * $squareHeight}]
set squareEndX [expr {$squareStartX + $squareWidth}]
set squareEndY [expr {$squareStartY + $squareHeight}]
set boundary "{{$squareStartX $squareStartY} {$squareEndX $squareEndY}}"
puts "方块 [$i,$j] 的boundary为:$boundary"
}
}
```
以上代码通过两层循环,分别控制横向和纵向的方块数量,然后根据当前方块的位置,通过计算得到每个方块的起始坐标和结束坐标,并将其整理成boundary的格式输出。输出的结果会显示每个方块的boundary。
### 回答3:
使用Tcl语言,可以使用以下代码将boundary为{{0 0}{1000 1000}}的区域分割成5x5的若干方块,并输出每个方块的boundary:
```tcl
set min_x 0
set min_y 0
set max_x 1000
set max_y 1000
set num_x 5
set num_y 5
set step_x [expr {($max_x - $min_x) / $num_x}]
set step_y [expr {($max_y - $min_y) / $num_y}]
for {set i 0} {$i < $num_x} {incr i} {
for {set j 0} {$j < $num_y} {incr j} {
set start_x [expr {$min_x + ($i * $step_x)}]
set start_y [expr {$min_y + ($j * $step_y)}]
set end_x [expr {$start_x + $step_x}]
set end_y [expr {$start_y + $step_y}]
set boundary "{{${start_x} ${start_y}} {${end_x} ${end_y}}}"
puts "方块 [$i,$j] 的boundary为 $boundary"
}
}
```
其中,min_x和min_y表示区域的左上角坐标,max_x和max_y表示区域的右下角坐标,num_x和num_y表示水平和垂直方向要分割的方块数量。
代码将使用嵌套的for循环来对区域进行分割,并计算每个方块的起始坐标和终止坐标。然后,将每个方块的boundary输出到控制台。
执行以上代码,将得到类似如下的结果:
```
方块 [0,0] 的boundary为 {{0 0} {200 200}}
方块 [0,1] 的boundary为 {{0 200} {200 400}}
方块 [0,2] 的boundary为 {{0 400} {200 600}}
方块 [0,3] 的boundary为 {{0 600} {200 800}}
方块 [0,4] 的boundary为 {{0 800} {200 1000}}
方块 [1,0] 的boundary为 {{200 0} {400 200}}
方块 [1,1] 的boundary为 {{200 200} {400 400}}
方块 [1,2] 的boundary为 {{200 400} {400 600}}
方块 [1,3] 的boundary为 {{200 600} {400 800}}
方块 [1,4] 的boundary为 {{200 800} {400 1000}}
方块 [2,0] 的boundary为 {{400 0} {600 200}}
方块 [2,1] 的boundary为 {{400 200} {600 400}}
方块 [2,2] 的boundary为 {{400 400} {600 600}}
方块 [2,3] 的boundary为 {{400 600} {600 800}}
方块 [2,4] 的boundary为 {{400 800} {600 1000}}
方块 [3,0] 的boundary为 {{600 0} {800 200}}
方块 [3,1] 的boundary为 {{600 200} {800 400}}
方块 [3,2] 的boundary为 {{600 400} {800 600}}
方块 [3,3] 的boundary为 {{600 600} {800 800}}
方块 [3,4] 的boundary为 {{600 800} {800 1000}}
方块 [4,0] 的boundary为 {{800 0} {1000 200}}
方块 [4,1] 的boundary为 {{800 200} {1000 400}}
方块 [4,2] 的boundary为 {{800 400} {1000 600}}
方块 [4,3] 的boundary为 {{800 600} {1000 800}}
方块 [4,4] 的boundary为 {{800 800} {1000 1000}}
```
阅读全文
相关推荐





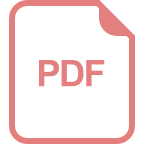




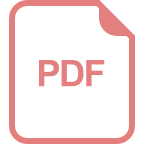




