前端怎么处理下载pdf文档的接口
时间: 2023-12-21 11:03:59 浏览: 32
前端可以通过发送一个GET或POST请求,将需要下载的PDF文档的URL地址传递给后端接口。后端接口可以使用一些流式处理的方式,将PDF文件的二进制数据流返回给前端。前端可以使用Blob对象或者FileSaver.js等库来处理二进制数据流并生成文件,实现下载功能。以下是一个使用fetch API下载PDF文档的示例代码:
```javascript
fetch('your-pdf-url')
.then(response => response.blob())
.then(blob => {
const url = window.URL.createObjectURL(new Blob([blob]));
const a = document.createElement('a');
a.href = url;
a.download = 'your-pdf-file-name';
document.body.appendChild(a);
a.click();
setTimeout(() => {
document.body.removeChild(a);
window.URL.revokeObjectURL(url);
}, 0);
});
```
其中,'your-pdf-url'是PDF文件的URL地址,'your-pdf-file-name'是将要下载的PDF文件的文件名。
相关问题
后端返回文件流给前端 PDF文件
可以在后端使用一些库或工具来生成 PDF 文件,例如 iText 或 Apache PDFBox。在生成完毕后,可以将生成的 PDF 文件以二进制流的形式返回给前端。
以下是一个使用 Spring Boot 框架返回 PDF 文件流的示例代码:
```java
@GetMapping("/download-pdf")
public ResponseEntity<ByteArrayResource> downloadPDF() throws IOException {
// 生成 PDF 文件
ByteArrayOutputStream baos = new ByteArrayOutputStream();
Document document = new Document();
PdfWriter.getInstance(document, baos);
document.open();
document.add(new Paragraph("Hello, World!"));
document.close();
// 将 PDF 文件以二进制流的形式返回给前端
ByteArrayResource resource = new ByteArrayResource(baos.toByteArray());
return ResponseEntity.ok()
.header(HttpHeaders.CONTENT_DISPOSITION, "attachment;filename=myfile.pdf")
.contentType(MediaType.APPLICATION_PDF)
.contentLength(resource.contentLength())
.body(resource);
}
```
这个示例代码会生成一个包含 "Hello, World!" 文本的 PDF 文件,并将其以二进制流的形式返回给前端。前端可以通过调用这个接口来下载 PDF 文件。
前端页面展示后台返回的pdf文件流
要在前端页面展示后台返回的 PDF 文件流,可以通过以下步骤实现:
1. 后台返回 PDF 文件流:确保后台接口正确地返回 PDF 文件的字节流。这通常可以通过使用合适的后台框架或库来实现。
2. 前端接收文件流:在前端使用 JavaScript,通过 AJAX 或 Fetch API 发起请求,获取后台返回的 PDF 文件流。可以将文件流保存到一个变量中。
3. 创建 Blob 对象:使用 JavaScript 的 Blob 对象,将文件流转换为可供浏览器解析的对象。Blob 对象代表了一个不可变的、原始数据的类文件对象。
```javascript
// 假设后台返回的文件流保存在 response 中
var blob = new Blob([response], { type: 'application/pdf' });
```
在上述代码中,我们使用 Blob 构造函数,将后台返回的文件流 response 转换为 Blob 对象,并指定 MIME 类型为 'application/pdf'。
4. 创建 URL 对象:使用 JavaScript 的 URL 对象,创建一个指向 Blob 对象的 URL。这个 URL 可以被用于在浏览器中打开或下载文件。
```javascript
var fileUrl = URL.createObjectURL(blob);
```
在上述代码中,我们使用 URL.createObjectURL() 函数,将 Blob 对象转换为 URL。这个 URL 可以被用于在浏览器中展示或下载文件。
5. 展示 PDF 文件:可以使用 `<iframe>` 元素或者 PDF.js 等技术在前端页面中展示 PDF 文件。
使用 `<iframe>` 展示 PDF 文件:
```html
<iframe src="fileUrl" width="100%" height="500px"></iframe>
```
在上述代码中,我们将前面创建的 fileUrl 设置为 `<iframe>` 的 src 属性,从而在页面中展示 PDF 文件。
使用 PDF.js 展示 PDF 文件:PDF.js 是一个开源的 JavaScript 库,可以在前端页面中渲染和展示 PDF 文件。您可以参考 PDF.js 的文档和示例来了解如何在页面中使用它。
通过上述步骤,您可以将后台返回的 PDF 文件流展示在前端页面中,并提供给用户查看或下载。请注意,这只是一种基本的实现方式,具体的实现可能会根据您使用的前端框架或库有所不同。
相关推荐
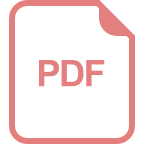












