c++ 删除一个目录下指定文件名的1.jpg和1.dat文件,不用c++17
时间: 2024-02-06 12:02:33 浏览: 43
如果您的编译器不支持C++17,您可以使用旧的文件操作函数来删除一个目录下指定文件名的1.jpg和1.dat文件。以下是一个示例代码:
```cpp
#include <iostream>
#include <string>
#include <dirent.h>
#include <unistd.h>
void deleteFiles(const std::string& directory, const std::string& filename) {
DIR* dir = opendir(directory.c_str());
if (dir == nullptr) {
std::cerr << "Failed to open directory: " << directory << std::endl;
return;
}
dirent* entry;
while ((entry = readdir(dir)) != nullptr) {
std::string file = entry->d_name;
if (file.find(filename) != std::string::npos && (file == "1.jpg" || file == "1.dat")) {
std::string filepath = directory + "/" + file;
if (unlink(filepath.c_str()) == 0) {
std::cout << "Deleted: " << filepath << std::endl;
} else {
std::cerr << "Failed to delete file: " << filepath << std::endl;
}
}
}
closedir(dir);
}
int main() {
std::string directory = "path/to/your/directory";
std::string filename = "1";
deleteFiles(directory, filename);
return 0;
}
```
您只需将代码中的 `"path/to/your/directory"` 替换为您想要删除文件的目录路径,将 `"1"` 替换为您指定的文件名。此代码会打开目录,遍历目录下的所有文件,检查文件名是否包含指定的文件名,并且文件名为"1.jpg"或"1.dat",如果是则删除该文件。
请注意,此代码使用了C语言的文件操作函数(opendir、readdir、unlink),并结合C++进行了封装。这种方法在早期的C++标准中是常用的文件操作方式。然而,这些函数在C++17中被新的文件系统库所取代,更推荐使用新的标准库来进行文件操作。
相关推荐
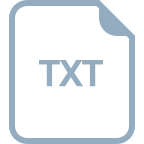
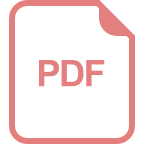














