在 Elasticsearch 中,size 和 from的用法,举个例子
时间: 2024-03-19 19:04:14 浏览: 9
在 Elasticsearch 中,size 和 from 是用来控制查询结果的数量和偏移量的参数。
- size:控制返回的结果数量,默认为10。
- from:控制返回结果的偏移量,默认为0。
以下是一个例子:
假设我们有一个名为 “blog” 的索引,其中包含了一些博客文章。我们想要查询最近发布的5篇文章,可以使用以下查询:
```
GET /blog/_search
{
"query": {
"match_all": {}
},
"sort": {
"publish_date": {
"order": "desc"
}
},
"size": 5,
"from": 0
}
```
在这个查询中,我们使用了 match_all 查询来匹配所有的文档,并按照 publish_date 字段的降序排序。我们还设置了 size 参数为5和 from 参数为0,以便返回最近发布的5篇文章。
相关问题
springboot2整合elasticsearch7,并生成elasticsearch工具类和生成调用例子
好的,我可以为您提供相关的信息和代码示例。首先,您需要确保您的Spring Boot项目中包含以下依赖项:
```xml
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-elasticsearch</artifactId>
</dependency>
<dependency>
<groupId>org.elasticsearch.client</groupId>
<artifactId>elasticsearch-rest-high-level-client</artifactId>
<version>7.0.0</version>
</dependency>
```
接下来,您需要在application.properties文件中添加以下配置:
```properties
spring.data.elasticsearch.cluster-name=my-application
spring.data.elasticsearch.cluster-nodes=localhost:9300
```
然后,您可以编写Elasticsearch工具类来执行一些常见的操作,例如索引文档、搜索文档等。以下是一个示例工具类:
```java
import org.elasticsearch.action.ActionListener;
import org.elasticsearch.action.bulk.BulkRequest;
import org.elasticsearch.action.bulk.BulkResponse;
import org.elasticsearch.action.get.GetRequest;
import org.elasticsearch.action.index.IndexRequest;
import org.elasticsearch.action.search.SearchRequest;
import org.elasticsearch.action.search.SearchResponse;
import org.elasticsearch.action.update.UpdateRequest;
import org.elasticsearch.client.RequestOptions;
import org.elasticsearch.client.RestHighLevelClient;
import org.elasticsearch.common.unit.TimeValue;
import org.elasticsearch.common.xcontent.XContentBuilder;
import org.elasticsearch.common.xcontent.XContentFactory;
import org.elasticsearch.index.query.QueryBuilders;
import org.elasticsearch.search.SearchHits;
import org.elasticsearch.search.builder.SearchSourceBuilder;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Component;
import java.io.IOException;
import java.util.Map;
@Component
public class ElasticsearchUtil {
@Autowired
private RestHighLevelClient restHighLevelClient;
/**
* 索引文档
* @param indexName 索引名称
* @param id 文档ID
* @param sourceMap 文档内容
* @throws IOException
*/
public void indexDocument(String indexName, String id, Map<String, Object> sourceMap) throws IOException {
IndexRequest request = new IndexRequest(indexName);
if (id != null) {
request.id(id);
}
request.source(sourceMap);
restHighLevelClient.index(request, RequestOptions.DEFAULT);
}
/**
* 更新文档
* @param indexName 索引名称
* @param id 文档ID
* @param sourceMap 文档内容
* @throws IOException
*/
public void updateDocument(String indexName, String id, Map<String, Object> sourceMap) throws IOException {
UpdateRequest request = new UpdateRequest(indexName, id);
request.doc(sourceMap);
restHighLevelClient.update(request, RequestOptions.DEFAULT);
}
/**
* 批量索引文档
* @param indexName 索引名称
* @param sourceMaps 文档内容列表
* @throws IOException
*/
public void bulkIndexDocuments(String indexName, Map<String, Object>[] sourceMaps) throws IOException {
BulkRequest request = new BulkRequest();
for (Map<String, Object> sourceMap : sourceMaps) {
IndexRequest indexRequest = new IndexRequest(indexName);
indexRequest.source(sourceMap);
request.add(indexRequest);
}
restHighLevelClient.bulk(request, RequestOptions.DEFAULT);
}
/**
* 批量更新文档
* @param indexName 索引名称
* @param idList 文档ID列表
* @param sourceMap 文档内容
* @throws IOException
*/
public void bulkUpdateDocuments(String indexName, String[] idList, Map<String, Object> sourceMap) throws IOException {
BulkRequest request = new BulkRequest();
for (String id : idList) {
UpdateRequest updateRequest = new UpdateRequest(indexName, id);
updateRequest.doc(sourceMap);
request.add(updateRequest);
}
restHighLevelClient.bulk(request, RequestOptions.DEFAULT);
}
/**
* 获取文档
* @param indexName 索引名称
* @param id 文档ID
* @return
* @throws IOException
*/
public Map<String, Object> getDocument(String indexName, String id) throws IOException {
GetRequest request = new GetRequest(indexName, id);
return restHighLevelClient.get(request, RequestOptions.DEFAULT).getSourceAsMap();
}
/**
* 搜索文档
* @param indexName 索引名称
* @param keyword 关键词
* @param size 每页数量
* @param from 起始位置
* @return
* @throws IOException
*/
public SearchHits searchDocuments(String indexName, String keyword, int size, int from) throws IOException {
SearchRequest searchRequest = new SearchRequest(indexName);
SearchSourceBuilder searchSourceBuilder = new SearchSourceBuilder();
searchSourceBuilder.query(QueryBuilders.matchQuery("content", keyword));
searchSourceBuilder.from(from);
searchSourceBuilder.size(size);
searchSourceBuilder.timeout(TimeValue.timeValueSeconds(60));
searchRequest.source(searchSourceBuilder);
SearchResponse searchResponse = restHighLevelClient.search(searchRequest, RequestOptions.DEFAULT);
return searchResponse.getHits();
}
}
```
最后,您可以在您的代码中使用ElasticsearchUtil类,并根据您的需求调用它的方法来执行所需的操作。以下是一个示例调用:
```java
import org.elasticsearch.search.SearchHits;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.bind.annotation.RestController;
import java.io.IOException;
import java.util.HashMap;
import java.util.Map;
@RestController
public class DemoController {
@Autowired
private ElasticsearchUtil elasticsearchUtil;
@GetMapping("/index")
public String index() throws IOException {
Map<String, Object> sourceMap = new HashMap<>();
sourceMap.put("title", "Elasticsearch 7 教程");
sourceMap.put("content", "Elasticsearch 7 入门教程");
elasticsearchUtil.indexDocument("my_index", null, sourceMap);
return "索引文档成功!";
}
@GetMapping("/search")
public String search(@RequestParam("keyword") String keyword,
@RequestParam("size") int size,
@RequestParam("from") int from) throws IOException {
SearchHits searchHits = elasticsearchUtil.searchDocuments("my_index", keyword, size, from);
return searchHits.toString();
}
}
```
希望这些代码示例可以帮助您整合Elasticsearch 7到您的Spring Boot项目中,并提供了一些常见的操作示例。
es搜索引擎bucket聚合的类型以及各自的用法,请各自举一个例子,并对案例进行逐条详解
好的,针对你的问题,我来为你解答一下。
在 Elasticsearch 搜索引擎中,bucket 聚合主要分为四种类型:terms、date histogram、range 和 geohash grid。
1. terms 聚合
terms 聚合是 Elasticsearch 中最常用的一种聚合方式,它会按照指定字段的值进行分组,并计算每个分组中的文档数量。这种聚合方式常用于统计某个字段的分布情况,例如商品分类、地区分布等。
举个例子,假设我们有一个电商网站,我们想要统计每个商品分类中的商品数量。那么我们可以使用 terms 聚合来实现:
```
GET /products/_search
{
"size": 0,
"aggs": {
"category": {
"terms": {
"field": "category.keyword"
}
}
}
}
```
在上面的例子中,我们通过 `terms` 聚合来统计 `category` 字段的分布情况。这里的 `keyword` 表示我们要对该字段进行精确匹配。最终返回的结果中,会按照每个分类进行分组,并统计每个分类中的商品数量。
2. date histogram 聚合
date histogram 聚合是针对日期类型的字段进行聚合,它会按照指定的时间间隔对时间字段进行分组,并计算每个时间段内的文档数量。这种聚合方式常用于统计某个时间段内的数据变化情况,例如每天的销售额、每月的用户活跃量等。
举个例子,假设我们有一个新闻网站,我们想要统计每天的新闻发布量。那么我们可以使用 date histogram 聚合来实现:
```
GET /news/_search
{
"size": 0,
"aggs": {
"daily": {
"date_histogram": {
"field": "publish_time",
"interval": "day"
}
}
}
}
```
在上面的例子中,我们通过 `date_histogram` 聚合来统计 `publish_time` 字段按天分布的情况。这里的 `interval` 表示我们要按照天来进行分组。最终返回的结果中,会按照每天进行分组,并统计每天的新闻发布量。
3. range 聚合
range 聚合是针对数值类型的字段进行聚合,它会按照指定的数值范围对字段进行分组,并计算每个数值范围内的文档数量。这种聚合方式常用于统计某个数值字段的分布情况,例如按照价格区间统计商品销量等。
举个例子,假设我们有一个电商网站,我们想要统计每个价格区间的商品数量。那么我们可以使用 range 聚合来实现:
```
GET /products/_search
{
"size": 0,
"aggs": {
"price_range": {
"range": {
"field": "price",
"ranges": [
{ "to": 10 },
{ "from": 10, "to": 100 },
{ "from": 100 }
]
}
}
}
}
```
在上面的例子中,我们通过 `range` 聚合来统计 `price` 字段的分布情况。这里的 `ranges` 表示我们要按照指定的数值范围来进行分组。最终返回的结果中,会按照每个价格区间进行分组,并统计每个价格区间内的商品数量。
4. geohash grid 聚合
geohash grid 聚合是针对地理位置类型的字段进行聚合,它会将地理位置划分为网格,并计算每个网格中的文档数量。这种聚合方式常用于统计某个地理区域内的数据分布情况,例如某个城市内的用户数量等。
举个例子,假设我们有一个城市的用户地理位置数据,我们想要统计每个网格内的用户数量。那么我们可以使用 geohash grid 聚合来实现:
```
GET /users/_search
{
"size": 0,
"aggs": {
"location": {
"geohash_grid": {
"field": "location",
"precision": 5
}
}
}
}
```
在上面的例子中,我们通过 `geohash_grid` 聚合来统计 `location` 字段的分布情况。这里的 `precision` 表示我们要将地理位置划分为多少个网格。最终返回的结果中,会按照每个网格进行分组,并统计每个网格内的用户数量。
以上就是 Elasticsearch 搜索引擎中四种常见的 bucket 聚合类型以及各自的用法和案例。希望能够对你有所帮助。
相关推荐
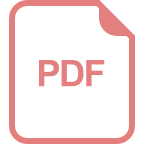
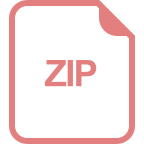
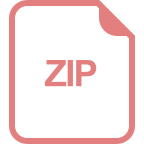
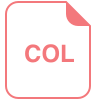
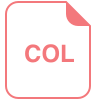
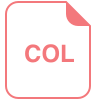
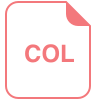
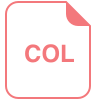







