找出无向图中所有环的julia代码
时间: 2023-09-23 17:06:25 浏览: 114
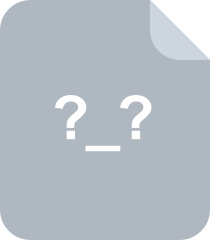
无向图中寻找所有的环路
### 回答1:
下面是一个简单的Julia代码,用于在无向图中找出所有环:
```julia
using LightGraphs
function find_cycles(g::AbstractGraph)
cycles = []
for node in vertices(g)
for neighbor in neighbors(g, node)
path = [node, neighbor]
current = neighbor
while current != node
next_node = nextneighbor(g, current, path[end])
if next_node == nothing
break
end
push!(path, next_node)
current = next_node
end
if current == node
push!(cycles, path)
end
end
end
return cycles
end
```
这段代码使用了LightGraphs包,它是一个用于处理图形数据的高效Julia库。上面的函数接受一个无向图(g)作为输入,并返回一个环的列表。首先,它对图中的每个节点进行遍历,然后对该节点的每个邻居进行遍历,如果当前节点和开始节点相同,则将路径添加到环的列表中。
### 回答2:
在Julia中,我们可以用图论库`LightGraphs`来找出无向图中的所有环。下面是使用`LightGraphs`库的代码示例:
```julia
using LightGraphs
# 创建一个无向图
graph = Graph(5) # 替换5为你想要的节点数量
# 添加边到图中
add_edge!(graph, 1, 2)
add_edge!(graph, 1, 3)
add_edge!(graph, 2, 3)
add_edge!(graph, 3, 4)
add_edge!(graph, 4, 5)
add_edge!(graph, 5, 1)
# 找出无向图中的所有环
cycles = find_cycle(graph)
# 输出所有环
for cycle in cycles
println(cycle)
end
```
在这个示例中,我们创建了一个有5个节点的无向图,然后添加了一些边。接着,我们使用`find_cycle`函数找出了所有的环,然后遍历并打印出每个环的节点。
注意:这只是一个示例,你可以根据需要修改图的大小、边的连接方式等。
### 回答3:
在Julia语言中,可以使用遍历算法来找出无向图中的所有环。以下是一个示例代码:
```julia
# 定义无向图
graph = Dict(
1 => [2, 3, 4],
2 => [1, 4],
3 => [1, 4],
4 => [1, 2, 3, 5],
5 => [4]
)
# 定义函数遍历无向图,查找所有环
function find_cycles(graph, start, node, visited, path, cycles)
visited[node] = true
push!(path, node)
for neighbor in graph[node]
if neighbor == start
push!(cycles, copy(path))
elseif !visited[neighbor]
find_cycles(graph, start, neighbor, visited, path, cycles)
end
end
pop!(path)
visited[node] = false
end
# 主函数,遍历无向图的每个节点
function find_all_cycles(graph)
cycles = []
for node in keys(graph)
visited = falses(length(graph))
path = []
find_cycles(graph, node, node, visited, path, cycles)
end
return cycles
end
# 调用主函数并输出结果
cycles = find_all_cycles(graph)
for cycle in cycles
println(cycle)
end
```
以上代码首先定义了一个无向图,其中`graph`是一个字典数据结构,表示节点之间的连接关系。然后,通过`find_cycles`函数使用深度优先搜索遍历无向图,查找所有环,并将它们存储在`cycles`数组中。最后,通过`find_all_cycles`函数遍历无向图的每个节点,调用`find_cycles`函数查找所有环,并将结果输出。
请注意,以上代码是一个简单示例,可以根据具体的无向图数据结构进行修改。
阅读全文
相关推荐
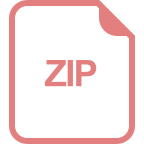
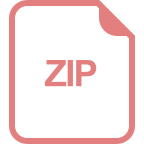
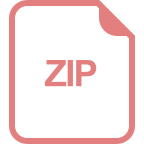
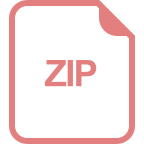
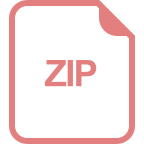
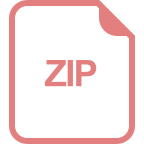
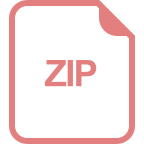
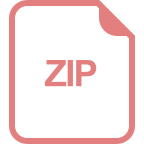
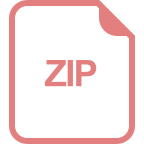
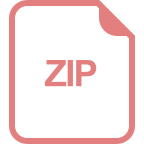
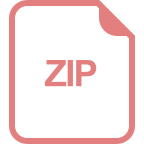
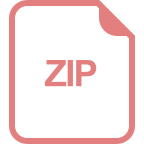
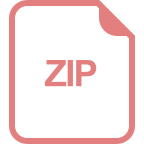
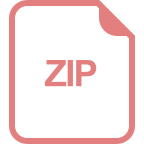
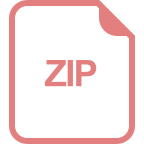
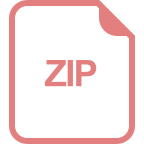