UnboundLocalError: local variable 'final_distance_positive' referenced before assignment
时间: 2023-10-23 07:08:35 浏览: 163
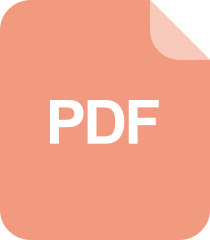
python UnboundLocalError: local variable ‘x’ referenced before assignment
This error occurs when a variable is accessed before being assigned a value within a local scope. Here is an example code that can produce this error:
```python
def calculate_distance(distance):
if distance > 0:
final_distance_positive = distance
return final_distance_positive
distance = 10
result = calculate_distance(distance)
print(result)
```
In this code, the function `calculate_distance()` checks if the input `distance` is positive. If it is, the variable `final_distance_positive` is assigned the value of `distance`. However, if `distance` is negative, the variable is never assigned a value. If the input is negative, the function will return an `UnboundLocalError` because `final_distance_positive` was never defined.
To fix this error, make sure to assign a default value to the variable outside of the conditional statement, or handle the negative condition properly. Here is an updated code that fixes this error:
```python
def calculate_distance(distance):
final_distance_positive = 0 # assign a default value
if distance > 0:
final_distance_positive = distance
return final_distance_positive
distance = 10
result = calculate_distance(distance)
print(result) # prints 10
distance = -10
result = calculate_distance(distance)
print(result) # prints 0
```
In this code, we assign a default value of 0 to `final_distance_positive` before the conditional statement. If the input `distance` is negative, the function will return the default value instead of throwing an error.
阅读全文
相关推荐
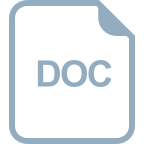
















